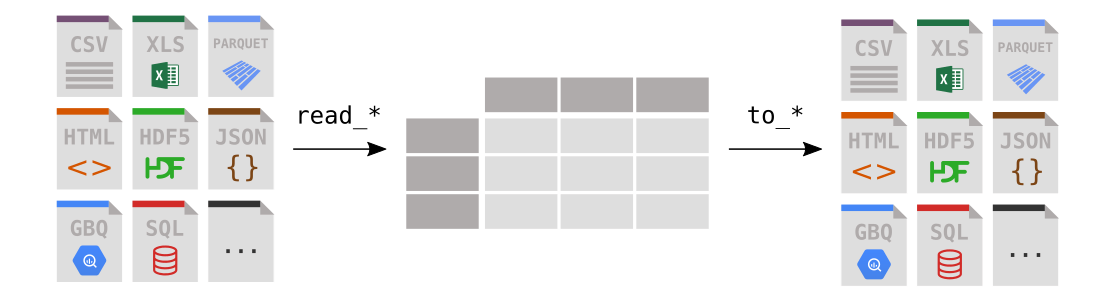
5 Reading Data
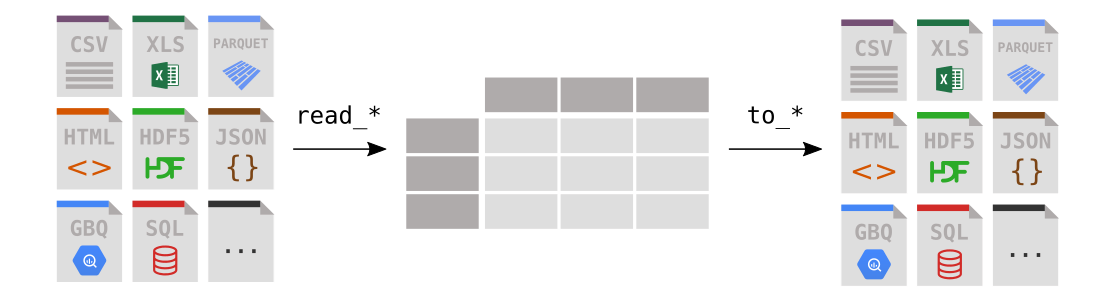
5.1 Types of data - structured and unstructured
Reading data is the first step to extract information from it. Data can exist broadly in two formats:
- Structured data, and
- Unstructured data.
Structured data is typically stored in a tabular form, where rows in the data correspond to “observations” and columns correspond to “variables”. For example, the following dataset contains 5 observations, where each observation (or row) consists of information about a movie. The variables (or columns) contain different pieces of information about a given movie. As all variables for a given row are related to the same movie, the data below is also called relational data.
Title | US Gross | Production Budget | Release Date | Major Genre | Creative Type | Rotten Tomatoes Rating | IMDB Rating | |
---|---|---|---|---|---|---|---|---|
0 | The Shawshank Redemption | 28241469 | 25000000 | Sep 23 1994 | Drama | Fiction | 88 | 9.2 |
1 | Inception | 285630280 | 160000000 | Jul 16 2010 | Horror/Thriller | Fiction | 87 | 9.1 |
2 | One Flew Over the Cuckoo's Nest | 108981275 | 4400000 | Nov 19 1975 | Comedy | Fiction | 96 | 8.9 |
3 | The Dark Knight | 533345358 | 185000000 | Jul 18 2008 | Action/Adventure | Fiction | 93 | 8.9 |
4 | Schindler's List | 96067179 | 25000000 | Dec 15 1993 | Drama | Non-Fiction | 97 | 8.9 |
Unstructured data is data that is not organized in any pre-defined manner. Examples of unstructured data can be text files, audio/video files, images, Internet of Things (IoT) data, etc. Unstructured data is relatively harder to analyze as most of the analytical methods and tools are oriented towards structured data. However, an unstructured data can be used to obtain structured data, which in turn can be analyzed. For example, an image can be converted to an array of pixels - which will be structured data. Machine learning algorithms can then be used on the array to classify the image as that of a dog or a cat.
In this course, we will focus on analyzing structured data.
Pandas is a popular Python library used for working in tabular data (similar to the data stored in a spreadsheet). Pandas provides helper functions to read data from various file formats like CSV, Excel spreadsheets, HTML tables, JSON, SQL, and more.
5.2 Reading a csv file with Pandas
Structured data can be stored in a variety of formats. The most popular format is data_file_name.csv, where the extension csv stands for comma separated values. The variable values of each observation are separated by a comma in a .csv file. In other words, the delimiter is a comma in a csv file. However, the comma is not visible when a .csv file is opened with Microsoft Excel.
The below csv file contains day-wise Covid-19 data for Italy:
date,new_cases,new_deaths,new_tests
2020-04-21,2256.0,454.0,28095.0
2020-04-22,2729.0,534.0,44248.0
2020-04-23,3370.0,437.0,37083.0
2020-04-24,2646.0,464.0,95273.0
2020-04-25,3021.0,420.0,38676.0
2020-04-26,2357.0,415.0,24113.0
2020-04-27,2324.0,260.0,26678.0
2020-04-28,1739.0,333.0,37554.0
...
CSVs: A comma-separated values (CSV) file is a delimited text file that uses a comma to separate values. Each line of the file is a data record. Each record consists of one or more fields, separated by commas. A CSV file typically stores tabular data (numbers and text) in plain text, in which case each line will have the same number of fields. (Wikipedia)
First, let’s import the Pandas library. As a convention, it is imported with the alias pd
.
import pandas as pd
import os
5.2.1 Using the read_csv
function
The pd.read_csv
function can be used to read a CSV file into a pandas DataFrame
: a spreadsheet-like object for analyzing and processing data.
= pd.read_csv('./datasets/movie_ratings.csv') movie_ratings
The built-in python function type
can be used to check the dataype of an object:
type(movie_ratings)
pandas.core.frame.DataFrame
We’ll learn more about DataFrame
in a future lesson.
Note that I use the relative path
to specify the file path for movie_ratings.csv, you may need to change it based on where you store the data file.
5.2.2 Data Overview
Once the data has been read, we may want to see what the data looks like. We’ll use another Pandas function head()
to view the first few rows of the data.
movie_ratings.head()
Title | US Gross | Worldwide Gross | Production Budget | Release Date | MPAA Rating | Source | Major Genre | Creative Type | IMDB Rating | IMDB Votes | |
---|---|---|---|---|---|---|---|---|---|---|---|
0 | Opal Dreams | 14443 | 14443 | 9000000 | Nov 22 2006 | PG/PG-13 | Adapted screenplay | Drama | Fiction | 6.5 | 468 |
1 | Major Dundee | 14873 | 14873 | 3800000 | Apr 07 1965 | PG/PG-13 | Adapted screenplay | Western/Musical | Fiction | 6.7 | 2588 |
2 | The Informers | 315000 | 315000 | 18000000 | Apr 24 2009 | R | Adapted screenplay | Horror/Thriller | Fiction | 5.2 | 7595 |
3 | Buffalo Soldiers | 353743 | 353743 | 15000000 | Jul 25 2003 | R | Adapted screenplay | Comedy | Fiction | 6.9 | 13510 |
4 | The Last Sin Eater | 388390 | 388390 | 2200000 | Feb 09 2007 | PG/PG-13 | Adapted screenplay | Drama | Fiction | 5.7 | 1012 |
Row Indices and column names (axis labels)
By default, when you create a pandas DataFrame (or Series) without specifying an index, pandas will automatically assign integer-based row indices starting from 0. These indices serve as the row labels and uniquely identify each row in the DataFrame. For example, the index 2 correponds to the row of the movie The Informers. By default, the indices are integers starting from 0. However, they can be changed (to even non-integer values) if desired by the user.
The bold text on top of the DataFrame refers to column names. For example, the column US Gross
consists of the gross revenue of a movie in the US.
Collectively, the indices and column names are referred as axis labels.
Basic information We can view some basic information about the data frame using the .info
method.
movie_ratings.info()
<class 'pandas.core.frame.DataFrame'>
RangeIndex: 2228 entries, 0 to 2227
Data columns (total 11 columns):
# Column Non-Null Count Dtype
--- ------ -------------- -----
0 Title 2228 non-null object
1 US Gross 2228 non-null int64
2 Worldwide Gross 2228 non-null int64
3 Production Budget 2228 non-null int64
4 Release Date 2228 non-null object
5 MPAA Rating 2228 non-null object
6 Source 2228 non-null object
7 Major Genre 2228 non-null object
8 Creative Type 2228 non-null object
9 IMDB Rating 2228 non-null float64
10 IMDB Votes 2228 non-null int64
dtypes: float64(1), int64(4), object(6)
memory usage: 191.6+ KB
The shape
property of a pandas DataFrame provides a tuple that represents the dimensions of the DataFrame:
- The first value in the tuple is the number of rows.
- The second value in the tuple is the number of columns.
movie_ratings.shape
(2228, 11)
The columns
property contains the list of columns within the data frame.
movie_ratings.columns
Index(['Title', 'US Gross', 'Worldwide Gross', 'Production Budget',
'Release Date', 'MPAA Rating', 'Source', 'Major Genre', 'Creative Type',
'IMDB Rating', 'IMDB Votes'],
dtype='object')
You can view statistical information for numerical columns (mean, standard deviation, minimum/maximum values, and the number of non-empty values) using the .describe
method.
movie_ratings.describe()
US Gross | Worldwide Gross | Production Budget | IMDB Rating | IMDB Votes | |
---|---|---|---|---|---|
count | 2.228000e+03 | 2.228000e+03 | 2.228000e+03 | 2228.000000 | 2228.000000 |
mean | 5.076370e+07 | 1.019370e+08 | 3.816055e+07 | 6.239004 | 33585.154847 |
std | 6.643081e+07 | 1.648589e+08 | 3.782604e+07 | 1.243285 | 47325.651561 |
min | 0.000000e+00 | 8.840000e+02 | 2.180000e+02 | 1.400000 | 18.000000 |
25% | 9.646188e+06 | 1.320737e+07 | 1.200000e+07 | 5.500000 | 6659.250000 |
50% | 2.838649e+07 | 4.266892e+07 | 2.600000e+07 | 6.400000 | 18169.000000 |
75% | 6.453140e+07 | 1.200000e+08 | 5.300000e+07 | 7.100000 | 40092.750000 |
max | 7.601676e+08 | 2.767891e+09 | 3.000000e+08 | 9.200000 | 519541.000000 |
Functions & Methods we’ve looked so far
pd.read_csv
- Read data from a CSV file into a PandasDataFrame
object.info()
- View basic infomation about rows, columns & data types.shape
- Get the number of rows & columns as a tuple.columns
- Get the list of column names.describe()
- View statistical information about numeric columns
5.3 Data Selection and Filtering
5.3.1 Extracting Column(s)
The first step when working with a DataFrame is often to extract one or more columns. To do this effectively, it’s helpful to understand the internal structure of a DataFrame. Conceptually, you can think of a DataFrame as a dictionary of lists, where the keys are column names, and the values are lists or arrays containing data for the respective columns.
# Pandas format is simliar to this
= {
movie_ratings_dict 'Title': ['Opal Dreams', 'Major Dundee', 'The Informers', 'Buffalo Soldiers', 'The Last Sin Eater'],
'US Gross': [14443, 14873, 315000, 353743, 388390],
'Worldwide Gross': [14443, 14873, 315000, 353743, 388390],
'Production Budget': [9000000, 3800000, 18000000, 15000000, 2200000]
}
For dictionary, we use key to retrive its values
'Title'] movie_ratings_dict[
['Opal Dreams',
'Major Dundee',
'The Informers',
'Buffalo Soldiers',
'The Last Sin Eater']
Similar like dictionary, we can extract a column by its column name
'Title'] movie_ratings[
0 Opal Dreams
1 Major Dundee
2 The Informers
3 Buffalo Soldiers
4 The Last Sin Eater
...
2223 King Arthur
2224 Mulan
2225 Robin Hood
2226 Robin Hood: Prince of Thieves
2227 Spiceworld
Name: Title, Length: 2228, dtype: object
Each column is a feature of the dataframe, we can also use.
operator to extract a single column
movie_ratings.Title
0 Opal Dreams
1 Major Dundee
2 The Informers
3 Buffalo Soldiers
4 The Last Sin Eater
...
2223 King Arthur
2224 Mulan
2225 Robin Hood
2226 Robin Hood: Prince of Thieves
2227 Spiceworld
Name: Title, Length: 2228, dtype: object
When extracting multiple columns, you need to place the column names inside a list.
'Title', 'US Gross', 'Worldwide Gross' ]] movie_ratings[[
Title | US Gross | Worldwide Gross | |
---|---|---|---|
0 | Opal Dreams | 14443 | 14443 |
1 | Major Dundee | 14873 | 14873 |
2 | The Informers | 315000 | 315000 |
3 | Buffalo Soldiers | 353743 | 353743 |
4 | The Last Sin Eater | 388390 | 388390 |
... | ... | ... | ... |
2223 | King Arthur | 51877963 | 203877963 |
2224 | Mulan | 120620254 | 303500000 |
2225 | Robin Hood | 105269730 | 310885538 |
2226 | Robin Hood: Prince of Thieves | 165493908 | 390500000 |
2227 | Spiceworld | 29342592 | 56042592 |
2228 rows × 3 columns
5.3.2 Extracting Row(s)
5.3.2.1 Extracting based on a Single Condition or Multiple Conditions
In many cases, we need to filter rows based on specific conditions or a combination of multiple conditions. Next, let’s explore how to use these conditions effectively to extract rows that meet our criteria, whether it’s a single condition or multiple conditions combined
# extracting the rows that have IMDB Rating greater than 8
'IMDB Rating'] > 8] movie_ratings[movie_ratings[
Title | US Gross | Worldwide Gross | Production Budget | Release Date | MPAA Rating | Source | Major Genre | Creative Type | IMDB Rating | IMDB Votes | |
---|---|---|---|---|---|---|---|---|---|---|---|
21 | Gandhi, My Father | 240425 | 1375194 | 5000000 | Aug 03 2007 | Other | Adapted screenplay | Drama | Non-Fiction | 8.1 | 50881 |
56 | Ed Wood | 5828466 | 5828466 | 18000000 | Sep 30 1994 | R | Adapted screenplay | Comedy | Non-Fiction | 8.1 | 74171 |
67 | Requiem for a Dream | 3635482 | 7390108 | 4500000 | Oct 06 2000 | Other | Adapted screenplay | Drama | Fiction | 8.5 | 185226 |
164 | Trainspotting | 16501785 | 24000785 | 3100000 | Jul 19 1996 | R | Adapted screenplay | Drama | Fiction | 8.2 | 150483 |
181 | The Wizard of Oz | 28202232 | 28202232 | 2777000 | Aug 25 2039 | G | Adapted screenplay | Western/Musical | Fiction | 8.3 | 102795 |
... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... |
2090 | Finding Nemo | 339714978 | 867894287 | 94000000 | May 30 2003 | G | Original Screenplay | Action/Adventure | Fiction | 8.2 | 165006 |
2092 | Toy Story 3 | 410640665 | 1046340665 | 200000000 | Jun 18 2010 | G | Original Screenplay | Action/Adventure | Fiction | 8.9 | 67380 |
2094 | Avatar | 760167650 | 2767891499 | 237000000 | Dec 18 2009 | PG/PG-13 | Original Screenplay | Action/Adventure | Fiction | 8.3 | 261439 |
2130 | Scarface | 44942821 | 44942821 | 25000000 | Dec 09 1983 | Other | Adapted screenplay | Drama | Fiction | 8.2 | 152262 |
2194 | The Departed | 133311000 | 290539042 | 90000000 | Oct 06 2006 | R | Adapted screenplay | Drama | Fiction | 8.5 | 264148 |
97 rows × 11 columns
To combine multiple conditions in pandas, you need to use the &
(AND) and |
(OR) operators. Make sure to enclose each condition in parentheses () for clarity and to ensure proper evaluation order.
# extracting the rows that have IMDB Rating greater than 8 and US Gross less than 1000000
'IMDB Rating'] > 8) & (movie_ratings['US Gross'] < 1000000)] movie_ratings[(movie_ratings[
Title | US Gross | Worldwide Gross | Production Budget | Release Date | MPAA Rating | Source | Major Genre | Creative Type | IMDB Rating | IMDB Votes | |
---|---|---|---|---|---|---|---|---|---|---|---|
21 | Gandhi, My Father | 240425 | 1375194 | 5000000 | Aug 03 2007 | Other | Adapted screenplay | Drama | Non-Fiction | 8.1 | 50881 |
636 | Lake of Fire | 25317 | 25317 | 6000000 | Oct 03 2007 | Other | Adapted screenplay | Documentary | Non-Fiction | 8.4 | 1027 |
5.3.2.2 Negating Conditions with the ~
Operator
The tilde (~
) is used for negating boolean conditions in pandas, making it a useful tool for excluding specific values or rows.
In some cases, we may want to extract rows that do not meet a specific condition. To accomplish this, we can use the ~
operator, which negates a condition. This operator allows us to filter data by excluding rows that satisfy a particular condition, making it a powerful tool for refining our queries. Let’s explore how to use the ~
operator to negate conditions and select rows that do not meet our criteria
# Excluding the rows that have IMDB Rating that equals 8 using the tilde ~
~(movie_ratings['IMDB Rating'] == 8)] movie_ratings[
Title | US Gross | Worldwide Gross | Production Budget | Release Date | MPAA Rating | Source | Major Genre | Creative Type | IMDB Rating | IMDB Votes | |
---|---|---|---|---|---|---|---|---|---|---|---|
0 | Opal Dreams | 14443 | 14443 | 9000000 | Nov 22 2006 | PG/PG-13 | Adapted screenplay | Drama | Fiction | 6.5 | 468 |
1 | Major Dundee | 14873 | 14873 | 3800000 | Apr 07 1965 | PG/PG-13 | Adapted screenplay | Western/Musical | Fiction | 6.7 | 2588 |
2 | The Informers | 315000 | 315000 | 18000000 | Apr 24 2009 | R | Adapted screenplay | Horror/Thriller | Fiction | 5.2 | 7595 |
3 | Buffalo Soldiers | 353743 | 353743 | 15000000 | Jul 25 2003 | R | Adapted screenplay | Comedy | Fiction | 6.9 | 13510 |
4 | The Last Sin Eater | 388390 | 388390 | 2200000 | Feb 09 2007 | PG/PG-13 | Adapted screenplay | Drama | Fiction | 5.7 | 1012 |
... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... |
2223 | King Arthur | 51877963 | 203877963 | 90000000 | Jul 07 2004 | PG/PG-13 | Adapted screenplay | Action/Adventure | Fiction | 6.2 | 53106 |
2224 | Mulan | 120620254 | 303500000 | 90000000 | Jun 19 1998 | G | Adapted screenplay | Action/Adventure | Non-Fiction | 7.2 | 34256 |
2225 | Robin Hood | 105269730 | 310885538 | 210000000 | May 14 2010 | PG/PG-13 | Adapted screenplay | Action/Adventure | Fiction | 6.9 | 34501 |
2226 | Robin Hood: Prince of Thieves | 165493908 | 390500000 | 50000000 | Jun 14 1991 | PG/PG-13 | Adapted screenplay | Action/Adventure | Fiction | 6.7 | 54480 |
2227 | Spiceworld | 29342592 | 56042592 | 25000000 | Jan 23 1998 | PG/PG-13 | Adapted screenplay | Comedy | Fiction | 2.9 | 18010 |
2191 rows × 11 columns
Another way to exclude rows where a column equals a certain value, you can use !=
to create the condition.
# using the != operator
'IMDB Rating'] != 8] movie_ratings[movie_ratings[
Title | US Gross | Worldwide Gross | Production Budget | Release Date | MPAA Rating | Source | Major Genre | Creative Type | IMDB Rating | IMDB Votes | |
---|---|---|---|---|---|---|---|---|---|---|---|
0 | Opal Dreams | 14443 | 14443 | 9000000 | Nov 22 2006 | PG/PG-13 | Adapted screenplay | Drama | Fiction | 6.5 | 468 |
1 | Major Dundee | 14873 | 14873 | 3800000 | Apr 07 1965 | PG/PG-13 | Adapted screenplay | Western/Musical | Fiction | 6.7 | 2588 |
2 | The Informers | 315000 | 315000 | 18000000 | Apr 24 2009 | R | Adapted screenplay | Horror/Thriller | Fiction | 5.2 | 7595 |
3 | Buffalo Soldiers | 353743 | 353743 | 15000000 | Jul 25 2003 | R | Adapted screenplay | Comedy | Fiction | 6.9 | 13510 |
4 | The Last Sin Eater | 388390 | 388390 | 2200000 | Feb 09 2007 | PG/PG-13 | Adapted screenplay | Drama | Fiction | 5.7 | 1012 |
... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... |
2223 | King Arthur | 51877963 | 203877963 | 90000000 | Jul 07 2004 | PG/PG-13 | Adapted screenplay | Action/Adventure | Fiction | 6.2 | 53106 |
2224 | Mulan | 120620254 | 303500000 | 90000000 | Jun 19 1998 | G | Adapted screenplay | Action/Adventure | Non-Fiction | 7.2 | 34256 |
2225 | Robin Hood | 105269730 | 310885538 | 210000000 | May 14 2010 | PG/PG-13 | Adapted screenplay | Action/Adventure | Fiction | 6.9 | 34501 |
2226 | Robin Hood: Prince of Thieves | 165493908 | 390500000 | 50000000 | Jun 14 1991 | PG/PG-13 | Adapted screenplay | Action/Adventure | Fiction | 6.7 | 54480 |
2227 | Spiceworld | 29342592 | 56042592 | 25000000 | Jan 23 1998 | PG/PG-13 | Adapted screenplay | Comedy | Fiction | 2.9 | 18010 |
2191 rows × 11 columns
5.3.3 Extracting Subsets of Rows and Columns
Sometimes we may be interested in working with a subset of rows and columns of the data, instead of working with the entire dataset. The indexing operators loc and iloc provide a convenient way of selecting a subset of desired rows and columns.
Let us first sort the movie_ratings
data frame by IMDB Rating
.
= movie_ratings.sort_values(by = 'IMDB Rating', ascending = False)
movie_ratings_sorted movie_ratings_sorted.head()
Title | US Gross | Worldwide Gross | Production Budget | Release Date | MPAA Rating | Source | Major Genre | Creative Type | IMDB Rating | IMDB Votes | |
---|---|---|---|---|---|---|---|---|---|---|---|
182 | The Shawshank Redemption | 28241469 | 28241469 | 25000000 | Sep 23 1994 | R | Adapted screenplay | Drama | Fiction | 9.2 | 519541 |
2084 | Inception | 285630280 | 753830280 | 160000000 | Jul 16 2010 | PG/PG-13 | Original Screenplay | Horror/Thriller | Fiction | 9.1 | 188247 |
790 | Schindler's List | 96067179 | 321200000 | 25000000 | Dec 15 1993 | R | Adapted screenplay | Drama | Non-Fiction | 8.9 | 276283 |
1962 | Pulp Fiction | 107928762 | 212928762 | 8000000 | Oct 14 1994 | R | Original Screenplay | Drama | Fiction | 8.9 | 417703 |
561 | The Dark Knight | 533345358 | 1022345358 | 185000000 | Jul 18 2008 | PG/PG-13 | Adapted screenplay | Action/Adventure | Fiction | 8.9 | 465000 |
5.3.3.1 Subsetting the DataFrame by loc
The operator loc
uses axis labels (row indices and column names) to subset the data.
Let’s subset the title
, worldwide gross
, production budget
, and IMDB raring
of top 3 movies.
# Subsetting the DataFrame by loc - using axis labels
= movie_ratings_sorted.loc[[182,2084, 2092],[ 'Title', 'IMDB Rating', 'US Gross', 'Worldwide Gross', 'Production Budget']]
movies_subset movies_subset
Title | IMDB Rating | US Gross | Worldwide Gross | Production Budget | |
---|---|---|---|---|---|
182 | The Shawshank Redemption | 9.2 | 28241469 | 28241469 | 25000000 |
2084 | Inception | 9.1 | 285630280 | 753830280 | 160000000 |
2092 | Toy Story 3 | 8.9 | 410640665 | 1046340665 | 200000000 |
The :
symbol in .loc
is a slicing operator that represents a range or all elements in the specified dimension (rows or columns). Use :
alone to select all rows/columns, or with start/end points to slice specific parts of the DataFrame.
# Subsetting the DataFrame by loc - using axis labels. the colon is used to select all rows
= movie_ratings_sorted.loc[:,['Title','Worldwide Gross','Production Budget','IMDB Rating']]
movies_subset movies_subset
Title | Worldwide Gross | Production Budget | IMDB Rating | |
---|---|---|---|---|
182 | The Shawshank Redemption | 28241469 | 25000000 | 9.2 |
2084 | Inception | 753830280 | 160000000 | 9.1 |
790 | Schindler's List | 321200000 | 25000000 | 8.9 |
1962 | Pulp Fiction | 212928762 | 8000000 | 8.9 |
561 | The Dark Knight | 1022345358 | 185000000 | 8.9 |
... | ... | ... | ... | ... |
1051 | Glitter | 4273372 | 8500000 | 2.0 |
1495 | Disaster Movie | 34690901 | 20000000 | 1.7 |
1116 | Crossover | 7009668 | 5600000 | 1.7 |
805 | From Justin to Kelly | 4922166 | 12000000 | 1.6 |
1147 | Super Babies: Baby Geniuses 2 | 9109322 | 20000000 | 1.4 |
2228 rows × 4 columns
# Subsetting the DataFrame by loc - using axis labels. the colon is used to select a range of rows
= movie_ratings_sorted.loc[182:561,['Title','Worldwide Gross','Production Budget','IMDB Rating']]
movies_subset movies_subset
Title | Worldwide Gross | Production Budget | IMDB Rating | |
---|---|---|---|---|
182 | The Shawshank Redemption | 28241469 | 25000000 | 9.2 |
2084 | Inception | 753830280 | 160000000 | 9.1 |
790 | Schindler's List | 321200000 | 25000000 | 8.9 |
1962 | Pulp Fiction | 212928762 | 8000000 | 8.9 |
561 | The Dark Knight | 1022345358 | 185000000 | 8.9 |
Combining .loc
with condition(s) to extract specific rows and columns based on criteria
# extracting the rows that have IMDB Rating greater than 8 or US Gross less than 1000000, only extract the Title and IMDB Rating columns
'IMDB Rating'] > 8) & (movie_ratings['US Gross'] < 1000000)][['Title','IMDB Rating']]
movie_ratings[(movie_ratings[
#using loc to extract the rows that have IMDB Rating greater than 8 or US Gross less than 1000000, only extract the Title and IMDB Rating columns
'IMDB Rating'] > 8) & (movie_ratings['US Gross'] < 1000000),['Title','IMDB Rating']] movie_ratings.loc[(movie_ratings[
Title | IMDB Rating | |
---|---|---|
21 | Gandhi, My Father | 8.1 |
636 | Lake of Fire | 8.4 |
5.3.3.2 Subsetting the DataFrame by iloc
while iloc
uses the position of rows or columns, where position has values 0,1,2,3,…and so on, for rows from top to bottom and columns from left to right. In other words, the first row has position 0, the second row has position 1, the third row has position 2, and so on. Similarly, the first column from left has position 0, the second column from left has position 1, the third column from left has position 2, and so on.
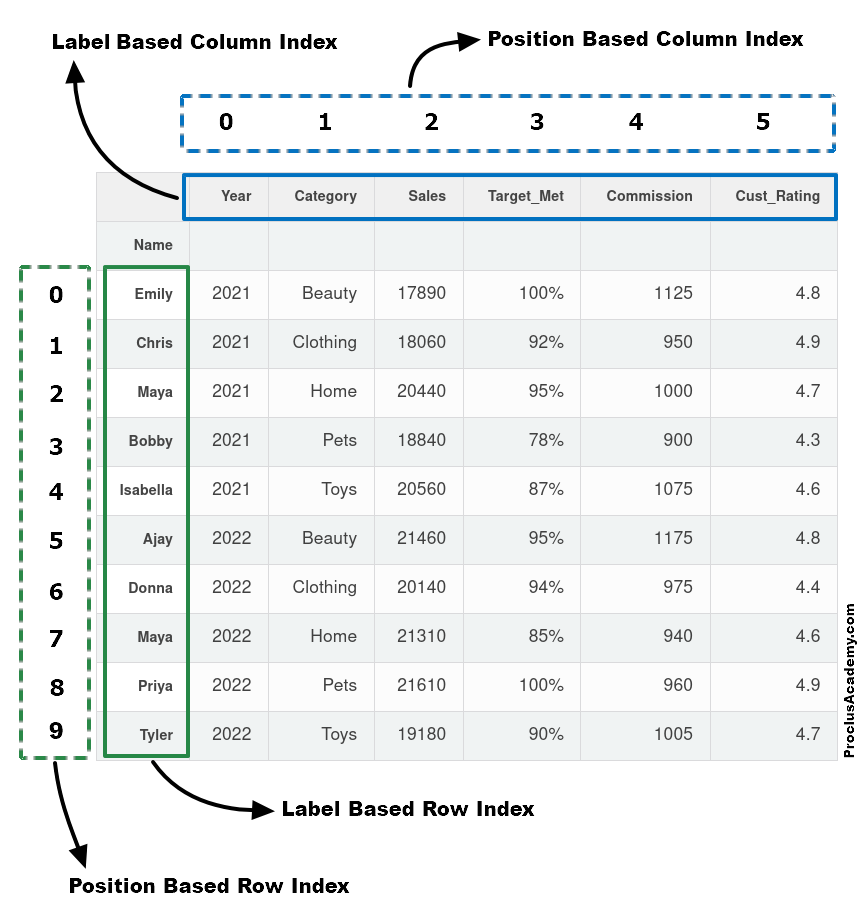
# let's check the movie_ratings_sorted DataFrame
movie_ratings_sorted.head()
Title | US Gross | Worldwide Gross | Production Budget | Release Date | MPAA Rating | Source | Major Genre | Creative Type | IMDB Rating | IMDB Votes | |
---|---|---|---|---|---|---|---|---|---|---|---|
182 | The Shawshank Redemption | 28241469 | 28241469 | 25000000 | Sep 23 1994 | R | Adapted screenplay | Drama | Fiction | 9.2 | 519541 |
2084 | Inception | 285630280 | 753830280 | 160000000 | Jul 16 2010 | PG/PG-13 | Original Screenplay | Horror/Thriller | Fiction | 9.1 | 188247 |
790 | Schindler's List | 96067179 | 321200000 | 25000000 | Dec 15 1993 | R | Adapted screenplay | Drama | Non-Fiction | 8.9 | 276283 |
1962 | Pulp Fiction | 107928762 | 212928762 | 8000000 | Oct 14 1994 | R | Original Screenplay | Drama | Fiction | 8.9 | 417703 |
561 | The Dark Knight | 533345358 | 1022345358 | 185000000 | Jul 18 2008 | PG/PG-13 | Adapted screenplay | Action/Adventure | Fiction | 8.9 | 465000 |
After sorting, the position-based index changes, while the label-based index remains unchanged. Let’s pass the position-based index to iloc
to retrieve the top 2 rows from the movie_ratings_sorted
DataFrame.
0:2,:] movie_ratings_sorted.iloc[
Title | US Gross | Worldwide Gross | Production Budget | Release Date | MPAA Rating | Source | Major Genre | Creative Type | IMDB Rating | IMDB Votes | |
---|---|---|---|---|---|---|---|---|---|---|---|
182 | The Shawshank Redemption | 28241469 | 28241469 | 25000000 | Sep 23 1994 | R | Adapted screenplay | Drama | Fiction | 9.2 | 519541 |
2084 | Inception | 285630280 | 753830280 | 160000000 | Jul 16 2010 | PG/PG-13 | Original Screenplay | Horror/Thriller | Fiction | 9.1 | 188247 |
It is important to note that the endpoint is excluded in an iloc
slice.
For comparison, let’s pass the same argument to loc and see what it returns.
0:2,:] movie_ratings_sorted.loc[
Title | US Gross | Worldwide Gross | Production Budget | Release Date | MPAA Rating | Source | Major Genre | Creative Type | IMDB Rating | IMDB Votes | |
---|---|---|---|---|---|---|---|---|---|---|---|
0 | Opal Dreams | 14443 | 14443 | 9000000 | Nov 22 2006 | PG/PG-13 | Adapted screenplay | Drama | Fiction | 6.5 | 468 |
851 | Star Trek: Generations | 75671262 | 120000000 | 38000000 | Nov 18 1994 | PG/PG-13 | Adapted screenplay | Action/Adventure | Fiction | 6.5 | 26465 |
140 | Tuck Everlasting | 19161999 | 19344615 | 15000000 | Oct 11 2002 | PG/PG-13 | Adapted screenplay | Drama | Fiction | 6.5 | 6639 |
708 | De-Lovely | 13337299 | 18396382 | 4000000 | Jun 25 2004 | PG/PG-13 | Adapted screenplay | Drama | Fiction | 6.5 | 6086 |
705 | Flyboys | 13090630 | 14816379 | 60000000 | Sep 22 2006 | PG/PG-13 | Adapted screenplay | Drama | Non-Fiction | 6.5 | 13934 |
... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... |
955 | The Brothers Solomon | 900926 | 900926 | 10000000 | Sep 07 2007 | R | Original Screenplay | Comedy | Fiction | 5.2 | 6044 |
1637 | Drumline | 56398162 | 56398162 | 20000000 | Dec 13 2002 | PG/PG-13 | Original Screenplay | Comedy | Fiction | 5.2 | 18165 |
1610 | Hollywood Homicide | 30207785 | 51107785 | 75000000 | Jun 13 2003 | PG/PG-13 | Original Screenplay | Action/Adventure | Fiction | 5.2 | 16452 |
569 | Doom | 28212337 | 54612337 | 70000000 | Oct 21 2005 | R | Adapted screenplay | Horror/Thriller | Fiction | 5.2 | 39473 |
2 | The Informers | 315000 | 315000 | 18000000 | Apr 24 2009 | R | Adapted screenplay | Horror/Thriller | Fiction | 5.2 | 7595 |
812 rows × 11 columns
As you can see, :
is used in the same way as in loc
to denote a range of the index. All rows between label indices 0 and 2, inclusive of both ends, are returned.
# Subsetting the DataFrame by iloc - using index of the position of rows and columns
= movie_ratings_sorted.iloc[0:10,[0,2,3,9]]
movies_iloc_subset1 movies_iloc_subset1
Title | Worldwide Gross | Production Budget | IMDB Rating | |
---|---|---|---|---|
182 | The Shawshank Redemption | 28241469 | 25000000 | 9.2 |
2084 | Inception | 753830280 | 160000000 | 9.1 |
2092 | Toy Story 3 | 1046340665 | 200000000 | 8.9 |
1962 | Pulp Fiction | 212928762 | 8000000 | 8.9 |
790 | Schindler's List | 321200000 | 25000000 | 8.9 |
561 | The Dark Knight | 1022345358 | 185000000 | 8.9 |
184 | Cidade de Deus | 28763397 | 3300000 | 8.8 |
487 | The Lord of the Rings: The Fellowship of the Ring | 868621686 | 109000000 | 8.8 |
497 | The Lord of the Rings: The Return of the King | 1133027325 | 94000000 | 8.8 |
1081 | C'era una volta il West | 5321508 | 5000000 | 8.8 |
Can you use .iloc
for conditional filtering, why or why not?
To recap, here are the Key differences betweenloc
and iloc
in pandas:
Indexing Type:
- loc uses labels (names) for indexing.
- iloc uses integer positions for indexing.
Inclusion of Endpoints:
- In a loc slice, both endpoints are included.
- In an iloc slice, the endpoint is excluded.
5.3.4 Finding minimum/maximum of a column
When working with pandas, there are two main options for locating the minimum or maximum values in a DataFrame column:
idxmin()
andidxmax()
: return the index label of the first occurrence of the maximum or minimum value in a specified column.
# movie_ratings_sorted.iloc[position_max_wgross,:]
= movie_ratings_sorted['Worldwide Gross'].idxmax()
max_index = movie_ratings_sorted['Worldwide Gross'].idxmin()
min_index print("Max index: ", max_index)
print("Min index: ", min_index)
Max index: 2094
Min index: 896
idxmin()
and idxmax()
return the index label of the minimum or maximum value in a column. You can use these returned index labels with .loc
to extract the corresponding row.
print(movie_ratings_sorted.loc[max_index,'Worldwide Gross'])
print(movie_ratings_sorted.loc[min_index,'Worldwide Gross'])
2767891499
884
argmax()
andargmin()
: Return the integer position of the first occurrence of the maximum or minimum value in a column. You can use these integer positions with.iloc
to extract the corresponding row
# using argmax and argmin, which return the index of the maximum and minimum values
= movie_ratings_sorted['Worldwide Gross'].argmax()
max_position = movie_ratings_sorted['Worldwide Gross'].argmin()
min_position print("max position:", max_position)
print("min position:", min_position)
# using iloc to get the row with the maximum and minimum values
print(movie_ratings_sorted.iloc[max_position, 2])
print(movie_ratings_sorted.iloc[min_position, 2])
max position: 48
min position: 2149
2767891499
884
Tips:
- When working with non-unique or custom indices, it is recommended to use
idxmax()
andidxmin()
to retrieve index labels, asargmax()
may be less intuitive in such scenarios.
- For DataFrames, use
.idxmax(axis=1)
or.idxmin(axis=1)
to find the index labels corresponding to the maximum or minimum values across rows, rather than columns.
5.3.5 Finding the top n minimum/maximum values of a column
To find the top n minimum or maximum values of a column in a pandas DataFrame, you can use the nsmallest()
and nlargest()
methods. Here’s how you can do it:
# find the top 3 movies with the highest worldwide gross
= movie_ratings.nlargest(3, 'Worldwide Gross')
top_3_movies top_3_movies
Title | US Gross | Worldwide Gross | Production Budget | Release Date | MPAA Rating | Source | Major Genre | Creative Type | IMDB Rating | IMDB Votes | |
---|---|---|---|---|---|---|---|---|---|---|---|
2094 | Avatar | 760167650 | 2767891499 | 237000000 | Dec 18 2009 | PG/PG-13 | Original Screenplay | Action/Adventure | Fiction | 8.3 | 261439 |
2093 | Titanic | 600788188 | 1842879955 | 200000000 | Dec 19 1997 | PG/PG-13 | Original Screenplay | Horror/Thriller | Fiction | 7.4 | 240732 |
497 | The Lord of the Rings: The Return of the King | 377027325 | 1133027325 | 94000000 | Dec 17 2003 | PG/PG-13 | Adapted screenplay | Action/Adventure | Fiction | 8.8 | 364077 |
Let’s double check the result using the movie_ratings_sorted
dataframe
'Worldwide Gross'].nlargest(3) movie_ratings_sorted[
2094 2767891499
2093 1842879955
497 1133027325
Name: Worldwide Gross, dtype: int64
Let’s find the 3 movies with the smallest IMDb votes using the nsmallest
method
= movie_ratings.nsmallest(3, 'IMDB Votes')
bottom_3_movies bottom_3_movies
Title | US Gross | Worldwide Gross | Production Budget | Release Date | MPAA Rating | Source | Major Genre | Creative Type | IMDB Rating | IMDB Votes | |
---|---|---|---|---|---|---|---|---|---|---|---|
1000 | Teeth | 347578 | 2300349 | 2000000 | Jan 18 2008 | R | Original Screenplay | Comedy | Fiction | 5.5 | 18 |
1261 | Birth | 5005899 | 14603001 | 20000000 | Oct 29 2004 | R | Original Screenplay | Drama | Fiction | 6.3 | 25 |
1298 | CachÈ | 3647381 | 17147381 | 8000000 | Dec 23 2005 | R | Original Screenplay | Drama | Fiction | 5.5 | 26 |
Let’s check the result using sort_values
method
# using the sort_values method
'IMDB Votes'].nsmallest(3) movie_ratings_sorted[
1000 18
1261 25
1298 26
Name: IMDB Votes, dtype: int64
5.4 Writing data to a .csv
file
The Pandas function to_csv
can be used to write (or export) data to a csv. Below is an example.
#Exporting the data of the top 250 movies to a csv file
'../data/movie_rating_exported.csv') movie_ratings.to_csv(
# check if the file has been exported
'../data') os.listdir(
['bestseller_books.txt',
'country-capital-lat-long-population.csv',
'covid.csv',
'fifa_data.csv',
'food_quantity.csv',
'gas_prices.csv',
'gdp_lifeExpectancy.csv',
'LOTR 2.csv',
'LOTR.csv',
'movies.csv',
'movies_cleaned.csv',
'movie_ratings.csv',
'movie_ratings.txt',
'movie_rating_exported.csv',
'party_nyc.csv',
'price.csv',
'question_json_data.json',
'spotify_data.csv',
'stocks.csv']
5.5 Reading other data formats - txt, html, json
Although .csv
is a very popular format for structured data, data is found in several other formats as well. Some of the other data formats are .txt
, .html
and .json
.
5.5.1 Reading .txt
files
The txt format offers some additional flexibility as compared to the csv format. In the csv format, the delimiter is a comma (or the column values are separated by a comma). However, in a txt file, the delimiter can be anything as desired by the user. Let us read the file movie_ratings.txt, where the variable values are separated by a tab character.
= pd.read_csv('../data/movie_ratings.txt',sep='\t')
movie_ratings_txt movie_ratings_txt.head()
Unnamed: 0 | Title | US Gross | Worldwide Gross | Production Budget | Release Date | MPAA Rating | Source | Major Genre | Creative Type | IMDB Rating | IMDB Votes | |
---|---|---|---|---|---|---|---|---|---|---|---|---|
0 | 0 | Opal Dreams | 14443 | 14443 | 9000000 | Nov 22 2006 | PG/PG-13 | Adapted screenplay | Drama | Fiction | 6.5 | 468 |
1 | 1 | Major Dundee | 14873 | 14873 | 3800000 | Apr 07 1965 | PG/PG-13 | Adapted screenplay | Western/Musical | Fiction | 6.7 | 2588 |
2 | 2 | The Informers | 315000 | 315000 | 18000000 | Apr 24 2009 | R | Adapted screenplay | Horror/Thriller | Fiction | 5.2 | 7595 |
3 | 3 | Buffalo Soldiers | 353743 | 353743 | 15000000 | Jul 25 2003 | R | Adapted screenplay | Comedy | Fiction | 6.9 | 13510 |
4 | 4 | The Last Sin Eater | 388390 | 388390 | 2200000 | Feb 09 2007 | PG/PG-13 | Adapted screenplay | Drama | Fiction | 5.7 | 1012 |
We use the function read_csv to read a txt file. However, we mention the tab character (r”) as a separator of variable values.
Note that there is no need to remember the argument name - sep for specifying the delimiter. You can always refer to the read_csv() documentation to find the relevant argument.
5.5.2 Reading HTML data
The Pandas function read_html searches for tabular data, i.e., data contained within the <table> tags of an html file. Let us read the tables in the GDP per capita page on Wikipedia.
#Reading all the tables from the Wikipedia page on GDP per capita
= pd.read_html('https://en.wikipedia.org/wiki/List_of_countries_by_GDP_(nominal)_per_capita') tables
All the tables will be read and stored in the variable named as tables. Let us find the datatype of the variable tables.
#Finidng datatype of the variable - tables
type(tables)
list
The variable - tables is a list of all the tables read from the HTML data.
#Number of tables read from the page
len(tables)
6
The in-built function len can be used to find the length of the list - tables or the number of tables read from the Wikipedia page. Let us check out the first table.
#Checking out the first table. Note that the index of the first table will be 0.
0] tables[
0 | 1 | 2 | |
---|---|---|---|
0 | >$60,000 $50,000–$60,000 $40,000–$50,000 $30,0... | $20,000–$30,000 $10,000–$20,000 $5,000–$10,000... | $1,000–$2,500 $500–$1,000 <$500 No data |
The above table doesn’t seem to be useful. Let us check out the second table.
#Checking out the second table. Note that the index of the first table will be 1.
1] tables[
Country/Territory | IMF[4][5] | World Bank[6] | United Nations[7] | ||||
---|---|---|---|---|---|---|---|
Country/Territory | Estimate | Year | Estimate | Year | Estimate | Year | |
0 | Monaco | — | — | 240862 | 2022 | 234317 | 2021 |
1 | Liechtenstein | — | — | 187267 | 2022 | 169260 | 2021 |
2 | Luxembourg | 131384 | 2024 | 128259 | 2023 | 133745 | 2021 |
3 | Bermuda | — | — | 123091 | 2022 | 112653 | 2021 |
4 | Ireland | 106059 | 2024 | 103685 | 2023 | 101109 | 2021 |
... | ... | ... | ... | ... | ... | ... | ... |
218 | Malawi | 481 | 2024 | 673 | 2023 | 613 | 2021 |
219 | South Sudan | 422 | 2024 | 1072 | 2015 | 400 | 2021 |
220 | Afghanistan | 422 | 2022 | 353 | 2022 | 373 | 2021 |
221 | Syria | — | — | 421 | 2021 | 925 | 2021 |
222 | Burundi | 230 | 2024 | 200 | 2023 | 311 | 2021 |
223 rows × 7 columns
The above table contains the estimated GDP per capita of all countries. This is the table that is likely to be relevant to a user interested in analyzing GDP per capita of countries. Instead of reading all tables of an HTML file, we can focus the search to tables containing certain relevant keywords. Let us try searching all table containing the word ‘Country’.
#Reading all the tables from the Wikipedia page on GDP per capita, containing the word 'Country'
= pd.read_html('https://en.wikipedia.org/wiki/List_of_countries_by_GDP_(nominal)_per_capita', match = 'Country') tables
The match argument can be used to specify the keywords to be present in the table to be read.
len(tables)
1
Only one table contains the keyword - ‘Country’. Let us check out the table obtained.
#Table having the keyword - 'Country' from the HTML page
0] tables[
Country/Territory | IMF[4][5] | World Bank[6] | United Nations[7] | ||||
---|---|---|---|---|---|---|---|
Country/Territory | Estimate | Year | Estimate | Year | Estimate | Year | |
0 | Monaco | — | — | 240862 | 2022 | 234317 | 2021 |
1 | Liechtenstein | — | — | 187267 | 2022 | 169260 | 2021 |
2 | Luxembourg | 131384 | 2024 | 128259 | 2023 | 133745 | 2021 |
3 | Bermuda | — | — | 123091 | 2022 | 112653 | 2021 |
4 | Ireland | 106059 | 2024 | 103685 | 2023 | 101109 | 2021 |
... | ... | ... | ... | ... | ... | ... | ... |
218 | Malawi | 481 | 2024 | 673 | 2023 | 613 | 2021 |
219 | South Sudan | 422 | 2024 | 1072 | 2015 | 400 | 2021 |
220 | Afghanistan | 422 | 2022 | 353 | 2022 | 373 | 2021 |
221 | Syria | — | — | 421 | 2021 | 925 | 2021 |
222 | Burundi | 230 | 2024 | 200 | 2023 | 311 | 2021 |
223 rows × 7 columns
The argument match helps with a more focussed search, and helps us discard irrelevant tables.
5.5.3 Reading JSON data
JSON stands for JavaScript Object Notation, in which the data is stored and transmitted as plain text. A couple of benefits of the JSON format are:
Since the format is text only, JSON data can easily be exchanged between web applications, and used by any programming language.
Unlike the csv format, JSON supports a hierarchical data structure, and is easier to integrate with APIs.
The JSON format can support a hierachical data structure, as it is built on the following two data structures (Source: technical documentation):
- A collection of name/value pairs. In various languages, this is realized as an object, record, struct, dictionary, hash table, keyed list, or associative array.
- An ordered list of values. In most languages, this is realized as an array, vector, list, or sequence.
These are universal data structures. Virtually all modern programming languages support them in one form or another. It makes sense that a data format that is interchangeable with programming languages also be based on these structures.
The Pandas function read_json converts a JSON string to a Pandas DataFrame. The function dumps() of the json library converts a Python object to a JSON string.
Lets read the JSON data on Ted Talks.
= pd.read_json('https://raw.githubusercontent.com/cwkenwaysun/TEDmap/master/data/TED_Talks.json') tedtalks_data
tedtalks_data.head()
id | speaker | headline | URL | description | transcript_URL | month_filmed | year_filmed | event | duration | date_published | tags | newURL | date | views | rates | |
---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|
0 | 7 | David Pogue | Simplicity sells | http://www.ted.com/talks/view/id/7 | New York Times columnist David Pogue takes aim... | http://www.ted.com/talks/view/id/7/transcript?... | 2 | 2006 | TED2006 | 0:21:26 | 6/27/06 | simplicity,computers,software,interface design... | https://www.ted.com/talks/david_pogue_says_sim... | 2006-06-27 | 1646773 | [{'id': 7, 'name': 'Funny', 'count': 968}, {'i... |
1 | 6 | Craig Venter | Sampling the ocean's DNA | http://www.ted.com/talks/view/id/6 | Genomics pioneer Craig Venter takes a break fr... | http://www.ted.com/talks/view/id/6/transcript?... | 7 | 2005 | TEDGlobal 2005 | 0:16:51 | 2004/05/07 | biotech,invention,oceans,genetics,DNA,biology,... | https://www.ted.com/talks/craig_venter_on_dna_... | 2004-05-07 | 562625 | [{'id': 3, 'name': 'Courageous', 'count': 21},... |
2 | 4 | Burt Rutan | The real future of space exploration | http://www.ted.com/talks/view/id/4 | In this passionate talk, legendary spacecraft ... | http://www.ted.com/talks/view/id/4/transcript?... | 2 | 2006 | TED2006 | 0:19:37 | 10/25/06 | aircraft,flight,industrial design,NASA,rocket ... | https://www.ted.com/talks/burt_rutan_sees_the_... | 2006-10-25 | 2046869 | [{'id': 3, 'name': 'Courageous', 'count': 169}... |
3 | 3 | Ashraf Ghani | How to rebuild a broken state | http://www.ted.com/talks/view/id/3 | Ashraf Ghani's passionate and powerful 10-minu... | http://www.ted.com/talks/view/id/3/transcript?... | 7 | 2005 | TEDGlobal 2005 | 0:18:45 | 10/18/06 | corruption,poverty,economics,investment,milita... | https://www.ted.com/talks/ashraf_ghani_on_rebu... | 2006-10-18 | 814554 | [{'id': 3, 'name': 'Courageous', 'count': 140}... |
4 | 5 | Chris Bangle | Great cars are great art | http://www.ted.com/talks/view/id/5 | American designer Chris Bangle explains his ph... | http://www.ted.com/talks/view/id/5/transcript?... | 2 | 2002 | TED2002 | 0:20:04 | 2004/05/07 | cars,industrial design,transportation,inventio... | https://www.ted.com/talks/chris_bangle_says_gr... | 2004-05-07 | 870950 | [{'id': 1, 'name': 'Beautiful', 'count': 89}, ... |
[{'question': "What is the data type of values in the last column (named 'rates') of the above dataset on ted talks",
'type': 'multiple_choice',
'answers': [{'answer': 'list', 'correct': True, 'feedback': 'Correct!'},
{'answer': 'string',
'correct': False,
'feedback': 'Incorrect. Use the type function on the variable to find its datatype.'},
{'answer': 'numeric',
'correct': False,
'feedback': 'Incorrect. Use the type function on the variable to find its datatype.'},
{'answer': 'dictionary',
'correct': False,
'feedback': 'Incorrect. Use the type function on the variable to find its datatype.'}]}]
This JSON data contains nested structures, such as lists and dictionaries, which require a deeper understanding to effectively structure. We will address this in future lectures
5.5.4 Reading data from a URL in Python
This process typically involves using the requests
library, which allows you to send HTTP requests and handle responses easily.
You’ll need to install it using pip
We’ll use the CoinGecko API, which provides cryptocurrency market data. Here’s an example of how to retrieve current market data:
import requests
# Define the URL of the API
= 'https://api.coingecko.com/api/v3/coins/markets?vs_currency=usd'
url
# Send a GET request to the URL
= requests.get(url)
response
# Check if the request was successful
if response.status_code == 200:
# Parse the JSON data
= response.json()
data print(data)
else:
print(f"Failed to retrieve data: {response.status_code}")
[{'id': 'bitcoin', 'symbol': 'btc', 'name': 'Bitcoin', 'image': 'https://coin-images.coingecko.com/coins/images/1/large/bitcoin.png?1696501400', 'current_price': 62490, 'market_cap': 1235032675967, 'market_cap_rank': 1, 'fully_diluted_valuation': 1312231173412, 'total_volume': 34554624888, 'high_24h': 64500, 'low_24h': 62100, 'price_change_24h': -1170.466984852057, 'price_change_percentage_24h': -1.83862, 'market_cap_change_24h': -23150749922.800293, 'market_cap_change_percentage_24h': -1.84001, 'circulating_supply': 19764571.0, 'total_supply': 21000000.0, 'max_supply': 21000000.0, 'ath': 73738, 'ath_change_percentage': -15.21931, 'ath_date': '2024-03-14T07:10:36.635Z', 'atl': 67.81, 'atl_change_percentage': 92093.56211, 'atl_date': '2013-07-06T00:00:00.000Z', 'roi': None, 'last_updated': '2024-10-08T00:54:10.271Z'}, {'id': 'ethereum', 'symbol': 'eth', 'name': 'Ethereum', 'image': 'https://coin-images.coingecko.com/coins/images/279/large/ethereum.png?1696501628', 'current_price': 2434.1, 'market_cap': 293023922868, 'market_cap_rank': 2, 'fully_diluted_valuation': 293018397207, 'total_volume': 16968707061, 'high_24h': 2518.07, 'low_24h': 2405.58, 'price_change_24h': -53.64323369453086, 'price_change_percentage_24h': -2.1563, 'market_cap_change_24h': -6417354167.597656, 'market_cap_change_percentage_24h': -2.14311, 'circulating_supply': 120379113.24004, 'total_supply': 120376843.206498, 'max_supply': None, 'ath': 4878.26, 'ath_change_percentage': -50.02647, 'ath_date': '2021-11-10T14:24:19.604Z', 'atl': 0.432979, 'atl_change_percentage': 562938.84293, 'atl_date': '2015-10-20T00:00:00.000Z', 'roi': {'times': 51.08601756823208, 'currency': 'btc', 'percentage': 5108.601756823207}, 'last_updated': '2024-10-08T00:54:07.565Z'}, {'id': 'tether', 'symbol': 'usdt', 'name': 'Tether', 'image': 'https://coin-images.coingecko.com/coins/images/325/large/Tether.png?1696501661', 'current_price': 0.999711, 'market_cap': 119827577712, 'market_cap_rank': 3, 'fully_diluted_valuation': 119827577712, 'total_volume': 55698442555, 'high_24h': 1.004, 'low_24h': 0.99633, 'price_change_24h': -0.001198196616044811, 'price_change_percentage_24h': -0.11971, 'market_cap_change_24h': -23449752.259277344, 'market_cap_change_percentage_24h': -0.01957, 'circulating_supply': 119857245003.333, 'total_supply': 119857245003.333, 'max_supply': None, 'ath': 1.32, 'ath_change_percentage': -24.39102, 'ath_date': '2018-07-24T00:00:00.000Z', 'atl': 0.572521, 'atl_change_percentage': 74.73226, 'atl_date': '2015-03-02T00:00:00.000Z', 'roi': None, 'last_updated': '2024-10-08T00:54:12.212Z'}, {'id': 'binancecoin', 'symbol': 'bnb', 'name': 'BNB', 'image': 'https://coin-images.coingecko.com/coins/images/825/large/bnb-icon2_2x.png?1696501970', 'current_price': 568.59, 'market_cap': 82947258162, 'market_cap_rank': 4, 'fully_diluted_valuation': 82947258162, 'total_volume': 799134450, 'high_24h': 582.45, 'low_24h': 563.77, 'price_change_24h': -7.260884048894809, 'price_change_percentage_24h': -1.2609, 'market_cap_change_24h': -1068798750.590683, 'market_cap_change_percentage_24h': -1.27214, 'circulating_supply': 145887575.79, 'total_supply': 145887575.79, 'max_supply': 200000000.0, 'ath': 717.48, 'ath_change_percentage': -20.80519, 'ath_date': '2024-06-06T14:10:59.816Z', 'atl': 0.0398177, 'atl_change_percentage': 1426911.78115, 'atl_date': '2017-10-19T00:00:00.000Z', 'roi': None, 'last_updated': '2024-10-08T00:54:13.445Z'}, {'id': 'solana', 'symbol': 'sol', 'name': 'Solana', 'image': 'https://coin-images.coingecko.com/coins/images/4128/large/solana.png?1718769756', 'current_price': 144.62, 'market_cap': 67873121370, 'market_cap_rank': 5, 'fully_diluted_valuation': 84775812388, 'total_volume': 3237160849, 'high_24h': 151.52, 'low_24h': 143.59, 'price_change_24h': -4.597486919219733, 'price_change_percentage_24h': -3.081, 'market_cap_change_24h': -2171285003.411751, 'market_cap_change_percentage_24h': -3.09987, 'circulating_supply': 469265730.374483, 'total_supply': 586128687.109744, 'max_supply': None, 'ath': 259.96, 'ath_change_percentage': -44.35227, 'ath_date': '2021-11-06T21:54:35.825Z', 'atl': 0.500801, 'atl_change_percentage': 28785.99929, 'atl_date': '2020-05-11T19:35:23.449Z', 'roi': None, 'last_updated': '2024-10-08T00:54:11.774Z'}, {'id': 'usd-coin', 'symbol': 'usdc', 'name': 'USDC', 'image': 'https://coin-images.coingecko.com/coins/images/6319/large/usdc.png?1696506694', 'current_price': 0.99982, 'market_cap': 35336575331, 'market_cap_rank': 6, 'fully_diluted_valuation': 35336582362, 'total_volume': 7937781611, 'high_24h': 1.003, 'low_24h': 0.996693, 'price_change_24h': -0.000988042958259383, 'price_change_percentage_24h': -0.09872, 'market_cap_change_24h': -295654890.59819794, 'market_cap_change_percentage_24h': -0.82974, 'circulating_supply': 35344977832.893, 'total_supply': 35344984865.7762, 'max_supply': None, 'ath': 1.17, 'ath_change_percentage': -14.68676, 'ath_date': '2019-05-08T00:40:28.300Z', 'atl': 0.877647, 'atl_change_percentage': 13.99504, 'atl_date': '2023-03-11T08:02:13.981Z', 'roi': None, 'last_updated': '2024-10-08T00:54:12.579Z'}, {'id': 'ripple', 'symbol': 'xrp', 'name': 'XRP', 'image': 'https://coin-images.coingecko.com/coins/images/44/large/xrp-symbol-white-128.png?1696501442', 'current_price': 0.531761, 'market_cap': 30080966724, 'market_cap_rank': 7, 'fully_diluted_valuation': 53173544466, 'total_volume': 1488925609, 'high_24h': 0.54488, 'low_24h': 0.529092, 'price_change_24h': -0.006764730959881505, 'price_change_percentage_24h': -1.25616, 'market_cap_change_24h': -380244778.19135284, 'market_cap_change_percentage_24h': -1.24829, 'circulating_supply': 56564039920.0, 'total_supply': 99987161962.0, 'max_supply': 100000000000.0, 'ath': 3.4, 'ath_change_percentage': -84.34345, 'ath_date': '2018-01-07T00:00:00.000Z', 'atl': 0.00268621, 'atl_change_percentage': 19707.86295, 'atl_date': '2014-05-22T00:00:00.000Z', 'roi': None, 'last_updated': '2024-10-08T00:54:11.049Z'}, {'id': 'staked-ether', 'symbol': 'steth', 'name': 'Lido Staked Ether', 'image': 'https://coin-images.coingecko.com/coins/images/13442/large/steth_logo.png?1696513206', 'current_price': 2433.54, 'market_cap': 23774301241, 'market_cap_rank': 8, 'fully_diluted_valuation': 23774301241, 'total_volume': 55393440, 'high_24h': 2515.61, 'low_24h': 2405.33, 'price_change_24h': -52.13318341543163, 'price_change_percentage_24h': -2.09734, 'market_cap_change_24h': -622209584.8816986, 'market_cap_change_percentage_24h': -2.5504, 'circulating_supply': 9767026.36285491, 'total_supply': 9767026.36285491, 'max_supply': None, 'ath': 4829.57, 'ath_change_percentage': -49.57783, 'ath_date': '2021-11-10T14:40:47.256Z', 'atl': 482.9, 'atl_change_percentage': 404.28528, 'atl_date': '2020-12-22T04:08:21.854Z', 'roi': None, 'last_updated': '2024-10-08T00:54:10.810Z'}, {'id': 'dogecoin', 'symbol': 'doge', 'name': 'Dogecoin', 'image': 'https://coin-images.coingecko.com/coins/images/5/large/dogecoin.png?1696501409', 'current_price': 0.109138, 'market_cap': 15967242014, 'market_cap_rank': 9, 'fully_diluted_valuation': 15967846781, 'total_volume': 994792895, 'high_24h': 0.11513, 'low_24h': 0.108236, 'price_change_24h': -0.004391041308727209, 'price_change_percentage_24h': -3.86778, 'market_cap_change_24h': -629528269.0053463, 'market_cap_change_percentage_24h': -3.79308, 'circulating_supply': 146268586383.705, 'total_supply': 146274126383.705, 'max_supply': None, 'ath': 0.731578, 'ath_change_percentage': -85.08287, 'ath_date': '2021-05-08T05:08:23.458Z', 'atl': 8.69e-05, 'atl_change_percentage': 125476.21875, 'atl_date': '2015-05-06T00:00:00.000Z', 'roi': None, 'last_updated': '2024-10-08T00:54:07.023Z'}, {'id': 'tron', 'symbol': 'trx', 'name': 'TRON', 'image': 'https://coin-images.coingecko.com/coins/images/1094/large/tron-logo.png?1696502193', 'current_price': 0.156189, 'market_cap': 13526695367, 'market_cap_rank': 10, 'fully_diluted_valuation': 13526702713, 'total_volume': 382377142, 'high_24h': 0.156897, 'low_24h': 0.153597, 'price_change_24h': 0.00148677, 'price_change_percentage_24h': 0.96105, 'market_cap_change_24h': 119102470, 'market_cap_change_percentage_24h': 0.88832, 'circulating_supply': 86577032111.108, 'total_supply': 86577079124.0223, 'max_supply': None, 'ath': 0.231673, 'ath_change_percentage': -32.53334, 'ath_date': '2018-01-05T00:00:00.000Z', 'atl': 0.00180434, 'atl_change_percentage': 8562.54313, 'atl_date': '2017-11-12T00:00:00.000Z', 'roi': {'times': 81.20479655054011, 'currency': 'usd', 'percentage': 8120.47965505401}, 'last_updated': '2024-10-08T00:54:05.672Z'}, {'id': 'the-open-network', 'symbol': 'ton', 'name': 'Toncoin', 'image': 'https://coin-images.coingecko.com/coins/images/17980/large/photo_2024-09-10_17.09.00.jpeg?1725963446', 'current_price': 5.23, 'market_cap': 13266378590, 'market_cap_rank': 11, 'fully_diluted_valuation': 26745582651, 'total_volume': 289898643, 'high_24h': 5.38, 'low_24h': 5.19, 'price_change_24h': -0.11581596106146907, 'price_change_percentage_24h': -2.1656, 'market_cap_change_24h': -292964162.0925293, 'market_cap_change_percentage_24h': -2.16061, 'circulating_supply': 2536031815.95331, 'total_supply': 5112747844.44798, 'max_supply': None, 'ath': 8.25, 'ath_change_percentage': -36.61515, 'ath_date': '2024-06-15T00:36:51.509Z', 'atl': 0.519364, 'atl_change_percentage': 907.29703, 'atl_date': '2021-09-21T00:33:11.092Z', 'roi': None, 'last_updated': '2024-10-08T00:54:13.375Z'}, {'id': 'cardano', 'symbol': 'ada', 'name': 'Cardano', 'image': 'https://coin-images.coingecko.com/coins/images/975/large/cardano.png?1696502090', 'current_price': 0.35311, 'market_cap': 12609866405, 'market_cap_rank': 12, 'fully_diluted_valuation': 15891641222, 'total_volume': 323896654, 'high_24h': 0.36763, 'low_24h': 0.350159, 'price_change_24h': -0.007715970856781273, 'price_change_percentage_24h': -2.13842, 'market_cap_change_24h': -274870486.73763466, 'market_cap_change_percentage_24h': -2.1333, 'circulating_supply': 35707072684.8988, 'total_supply': 45000000000.0, 'max_supply': 45000000000.0, 'ath': 3.09, 'ath_change_percentage': -88.54235, 'ath_date': '2021-09-02T06:00:10.474Z', 'atl': 0.01925275, 'atl_change_percentage': 1737.07582, 'atl_date': '2020-03-13T02:22:55.044Z', 'roi': None, 'last_updated': '2024-10-08T00:54:06.319Z'}, {'id': 'avalanche-2', 'symbol': 'avax', 'name': 'Avalanche', 'image': 'https://coin-images.coingecko.com/coins/images/12559/large/Avalanche_Circle_RedWhite_Trans.png?1696512369', 'current_price': 26.86, 'market_cap': 10918471696, 'market_cap_rank': 13, 'fully_diluted_valuation': 11993041109, 'total_volume': 383447410, 'high_24h': 27.69, 'low_24h': 26.52, 'price_change_24h': -0.30149710548148434, 'price_change_percentage_24h': -1.10995, 'market_cap_change_24h': -122018390.50128365, 'market_cap_change_percentage_24h': -1.10519, 'circulating_supply': 406462834.22799, 'total_supply': 446465917.213367, 'max_supply': 720000000.0, 'ath': 144.96, 'ath_change_percentage': -81.48341, 'ath_date': '2021-11-21T14:18:56.538Z', 'atl': 2.8, 'atl_change_percentage': 858.27418, 'atl_date': '2020-12-31T13:15:21.540Z', 'roi': None, 'last_updated': '2024-10-08T00:54:04.331Z'}, {'id': 'shiba-inu', 'symbol': 'shib', 'name': 'Shiba Inu', 'image': 'https://coin-images.coingecko.com/coins/images/11939/large/shiba.png?1696511800', 'current_price': 1.759e-05, 'market_cap': 10365613559, 'market_cap_rank': 14, 'fully_diluted_valuation': 17590632069, 'total_volume': 791497323, 'high_24h': 1.873e-05, 'low_24h': 1.746e-05, 'price_change_24h': -9.50643038621e-07, 'price_change_percentage_24h': -5.12756, 'market_cap_change_24h': -564457717.0176163, 'market_cap_change_percentage_24h': -5.16426, 'circulating_supply': 589258560734548.0, 'total_supply': 999982343215162.0, 'max_supply': None, 'ath': 8.616e-05, 'ath_change_percentage': -79.56469, 'ath_date': '2021-10-28T03:54:55.568Z', 'atl': 5.6366e-11, 'atl_change_percentage': 31236278.95797, 'atl_date': '2020-11-28T11:26:25.838Z', 'roi': None, 'last_updated': '2024-10-08T00:54:11.049Z'}, {'id': 'wrapped-steth', 'symbol': 'wsteth', 'name': 'Wrapped stETH', 'image': 'https://coin-images.coingecko.com/coins/images/18834/large/wstETH.png?1696518295', 'current_price': 2870.49, 'market_cap': 10083391703, 'market_cap_rank': 15, 'fully_diluted_valuation': 10083391703, 'total_volume': 57354973, 'high_24h': 2967.08, 'low_24h': 2856.16, 'price_change_24h': -65.57570573917383, 'price_change_percentage_24h': -2.23345, 'market_cap_change_24h': -183962777.72447205, 'market_cap_change_percentage_24h': -1.79173, 'circulating_supply': 3515218.76797872, 'total_supply': 3515218.76797872, 'max_supply': None, 'ath': 7256.02, 'ath_change_percentage': -60.4339, 'ath_date': '2022-05-13T15:09:54.509Z', 'atl': 558.54, 'atl_change_percentage': 414.00503, 'atl_date': '2022-05-13T01:36:45.053Z', 'roi': None, 'last_updated': '2024-10-08T00:54:12.928Z'}, {'id': 'wrapped-bitcoin', 'symbol': 'wbtc', 'name': 'Wrapped Bitcoin', 'image': 'https://coin-images.coingecko.com/coins/images/7598/large/wrapped_bitcoin_wbtc.png?1696507857', 'current_price': 62339, 'market_cap': 9370148390, 'market_cap_rank': 16, 'fully_diluted_valuation': 9370148390, 'total_volume': 173837802, 'high_24h': 64104, 'low_24h': 62051, 'price_change_24h': -1114.1613043298566, 'price_change_percentage_24h': -1.75588, 'market_cap_change_24h': -212142102.48479843, 'market_cap_change_percentage_24h': -2.2139, 'circulating_supply': 150281.33314858, 'total_supply': 150281.33314858, 'max_supply': 150281.33314858, 'ath': 73505, 'ath_change_percentage': -15.19126, 'ath_date': '2024-03-14T07:10:23.403Z', 'atl': 3139.17, 'atl_change_percentage': 1885.84407, 'atl_date': '2019-04-02T00:00:00.000Z', 'roi': None, 'last_updated': '2024-10-08T00:54:12.624Z'}, {'id': 'weth', 'symbol': 'weth', 'name': 'WETH', 'image': 'https://coin-images.coingecko.com/coins/images/2518/large/weth.png?1696503332', 'current_price': 2434.35, 'market_cap': 7231757765, 'market_cap_rank': 17, 'fully_diluted_valuation': 7231757765, 'total_volume': 421839130, 'high_24h': 2514.46, 'low_24h': 2412.75, 'price_change_24h': -52.1605917937668, 'price_change_percentage_24h': -2.09774, 'market_cap_change_24h': -167754303.50990772, 'market_cap_change_percentage_24h': -2.2671, 'circulating_supply': 2973433.46470016, 'total_supply': 2973433.46470016, 'max_supply': None, 'ath': 4799.89, 'ath_change_percentage': -49.27022, 'ath_date': '2021-11-09T00:00:00.000Z', 'atl': 82.1, 'atl_change_percentage': 2865.74184, 'atl_date': '2018-12-15T00:00:00.000Z', 'roi': None, 'last_updated': '2024-10-08T00:54:04.743Z'}, {'id': 'chainlink', 'symbol': 'link', 'name': 'Chainlink', 'image': 'https://coin-images.coingecko.com/coins/images/877/large/chainlink-new-logo.png?1696502009', 'current_price': 11.22, 'market_cap': 7034017951, 'market_cap_rank': 18, 'fully_diluted_valuation': 11221214442, 'total_volume': 429742466, 'high_24h': 11.74, 'low_24h': 11.11, 'price_change_24h': -0.20415057957979954, 'price_change_percentage_24h': -1.78697, 'market_cap_change_24h': -127373629.90058899, 'market_cap_change_percentage_24h': -1.77862, 'circulating_supply': 626849971.3083414, 'total_supply': 1000000000.0, 'max_supply': 1000000000.0, 'ath': 52.7, 'ath_change_percentage': -78.69639, 'ath_date': '2021-05-10T00:13:57.214Z', 'atl': 0.148183, 'atl_change_percentage': 7475.94449, 'atl_date': '2017-11-29T00:00:00.000Z', 'roi': None, 'last_updated': '2024-10-08T00:54:05.960Z'}, {'id': 'bitcoin-cash', 'symbol': 'bch', 'name': 'Bitcoin Cash', 'image': 'https://coin-images.coingecko.com/coins/images/780/large/bitcoin-cash-circle.png?1696501932', 'current_price': 325.66, 'market_cap': 6438369641, 'market_cap_rank': 19, 'fully_diluted_valuation': 6838542455, 'total_volume': 245664732, 'high_24h': 332.33, 'low_24h': 321.68, 'price_change_24h': -2.1671119914790893, 'price_change_percentage_24h': -0.66106, 'market_cap_change_24h': -44835556.588739395, 'market_cap_change_percentage_24h': -0.69156, 'circulating_supply': 19771137.3966508, 'total_supply': 21000000.0, 'max_supply': 21000000.0, 'ath': 3785.82, 'ath_change_percentage': -91.40438, 'ath_date': '2017-12-20T00:00:00.000Z', 'atl': 76.93, 'atl_change_percentage': 322.97489, 'atl_date': '2018-12-16T00:00:00.000Z', 'roi': None, 'last_updated': '2024-10-08T00:54:07.631Z'}, {'id': 'polkadot', 'symbol': 'dot', 'name': 'Polkadot', 'image': 'https://coin-images.coingecko.com/coins/images/12171/large/polkadot.png?1696512008', 'current_price': 4.15, 'market_cap': 5904726419, 'market_cap_rank': 20, 'fully_diluted_valuation': 6240862690, 'total_volume': 179496820, 'high_24h': 4.27, 'low_24h': 4.13, 'price_change_24h': -0.061744048750748355, 'price_change_percentage_24h': -1.46434, 'market_cap_change_24h': -89644363.9776411, 'market_cap_change_percentage_24h': -1.49548, 'circulating_supply': 1421990197.81355, 'total_supply': 1502939330.71355, 'max_supply': None, 'ath': 54.98, 'ath_change_percentage': -92.44717, 'ath_date': '2021-11-04T14:10:09.301Z', 'atl': 2.7, 'atl_change_percentage': 53.94571, 'atl_date': '2020-08-20T05:48:11.359Z', 'roi': None, 'last_updated': '2024-10-08T00:54:04.800Z'}, {'id': 'dai', 'symbol': 'dai', 'name': 'Dai', 'image': 'https://coin-images.coingecko.com/coins/images/9956/large/Badge_Dai.png?1696509996', 'current_price': 0.999811, 'market_cap': 5881292215, 'market_cap_rank': 21, 'fully_diluted_valuation': 5881798975, 'total_volume': 63258183, 'high_24h': 1.003, 'low_24h': 0.994743, 'price_change_24h': -0.000764124895492824, 'price_change_percentage_24h': -0.07637, 'market_cap_change_24h': 3577027, 'market_cap_change_percentage_24h': 0.06086, 'circulating_supply': 5882619286.90039, 'total_supply': 5883126161.262, 'max_supply': None, 'ath': 1.22, 'ath_change_percentage': -17.94271, 'ath_date': '2020-03-13T03:02:50.373Z', 'atl': 0.88196, 'atl_change_percentage': 13.40894, 'atl_date': '2023-03-11T07:50:50.514Z', 'roi': None, 'last_updated': '2024-10-08T00:54:06.710Z'}, {'id': 'sui', 'symbol': 'sui', 'name': 'Sui', 'image': 'https://coin-images.coingecko.com/coins/images/26375/large/sui-ocean-square.png?1727791290', 'current_price': 2.06, 'market_cap': 5685791571, 'market_cap_rank': 22, 'fully_diluted_valuation': 20572061868, 'total_volume': 2077001293, 'high_24h': 2.14, 'low_24h': 1.87, 'price_change_24h': 0.154302, 'price_change_percentage_24h': 8.10887, 'market_cap_change_24h': 415591222, 'market_cap_change_percentage_24h': 7.88568, 'circulating_supply': 2763841372.60889, 'total_supply': 10000000000.0, 'max_supply': 10000000000.0, 'ath': 2.17, 'ath_change_percentage': -5.01098, 'ath_date': '2024-03-27T17:46:02.608Z', 'atl': 0.364846, 'atl_change_percentage': 465.24479, 'atl_date': '2023-10-19T10:40:30.078Z', 'roi': None, 'last_updated': '2024-10-08T00:54:04.265Z'}, {'id': 'near', 'symbol': 'near', 'name': 'NEAR Protocol', 'image': 'https://coin-images.coingecko.com/coins/images/10365/large/near.jpg?1696510367', 'current_price': 5.11, 'market_cap': 5660830269, 'market_cap_rank': 23, 'fully_diluted_valuation': 6049736931, 'total_volume': 576314968, 'high_24h': 5.31, 'low_24h': 5.01, 'price_change_24h': 0.095049, 'price_change_percentage_24h': 1.89681, 'market_cap_change_24h': 113909251, 'market_cap_change_percentage_24h': 2.05356, 'circulating_supply': 1107181322.94878, 'total_supply': 1183246170.6779, 'max_supply': None, 'ath': 20.44, 'ath_change_percentage': -75.01369, 'ath_date': '2022-01-16T22:09:45.873Z', 'atl': 0.526762, 'atl_change_percentage': 869.45631, 'atl_date': '2020-11-04T16:09:15.137Z', 'roi': None, 'last_updated': '2024-10-08T00:54:11.280Z'}, {'id': 'leo-token', 'symbol': 'leo', 'name': 'LEO Token', 'image': 'https://coin-images.coingecko.com/coins/images/8418/large/leo-token.png?1696508607', 'current_price': 6.01, 'market_cap': 5560203959, 'market_cap_rank': 24, 'fully_diluted_valuation': 5920434513, 'total_volume': 801407, 'high_24h': 6.05, 'low_24h': 5.93, 'price_change_24h': -0.027411011021039933, 'price_change_percentage_24h': -0.45384, 'market_cap_change_24h': -24784317.658293724, 'market_cap_change_percentage_24h': -0.44377, 'circulating_supply': 925292320.9, 'total_supply': 985239504.0, 'max_supply': None, 'ath': 8.14, 'ath_change_percentage': -25.84331, 'ath_date': '2022-02-08T17:40:10.285Z', 'atl': 0.799859, 'atl_change_percentage': 654.24544, 'atl_date': '2019-12-24T15:14:35.376Z', 'roi': None, 'last_updated': '2024-10-08T00:54:08.729Z'}, {'id': 'uniswap', 'symbol': 'uni', 'name': 'Uniswap', 'image': 'https://coin-images.coingecko.com/coins/images/12504/large/uniswap-logo.png?1720676669', 'current_price': 7.24, 'market_cap': 5464326017, 'market_cap_rank': 25, 'fully_diluted_valuation': 7249360122, 'total_volume': 231484762, 'high_24h': 7.46, 'low_24h': 7.17, 'price_change_24h': 0.0370806, 'price_change_percentage_24h': 0.5151, 'market_cap_change_24h': 40188389, 'market_cap_change_percentage_24h': 0.74092, 'circulating_supply': 753766667.0, 'total_supply': 1000000000.0, 'max_supply': 1000000000.0, 'ath': 44.92, 'ath_change_percentage': -83.84698, 'ath_date': '2021-05-03T05:25:04.822Z', 'atl': 1.03, 'atl_change_percentage': 604.31268, 'atl_date': '2020-09-17T01:20:38.214Z', 'roi': None, 'last_updated': '2024-10-08T00:54:12.072Z'}, {'id': 'litecoin', 'symbol': 'ltc', 'name': 'Litecoin', 'image': 'https://coin-images.coingecko.com/coins/images/2/large/litecoin.png?1696501400', 'current_price': 65.04, 'market_cap': 4881816975, 'market_cap_rank': 26, 'fully_diluted_valuation': 5463392544, 'total_volume': 332840383, 'high_24h': 67.87, 'low_24h': 64.44, 'price_change_24h': -2.5680815804710733, 'price_change_percentage_24h': -3.79825, 'market_cap_change_24h': -192122729.2591772, 'market_cap_change_percentage_24h': -3.78646, 'circulating_supply': 75058239.4834713, 'total_supply': 84000000.0, 'max_supply': 84000000.0, 'ath': 410.26, 'ath_change_percentage': -84.11656, 'ath_date': '2021-05-10T03:13:07.904Z', 'atl': 1.15, 'atl_change_percentage': 5572.09067, 'atl_date': '2015-01-14T00:00:00.000Z', 'roi': None, 'last_updated': '2024-10-08T00:54:09.994Z'}, {'id': 'bittensor', 'symbol': 'tao', 'name': 'Bittensor', 'image': 'https://coin-images.coingecko.com/coins/images/28452/large/ARUsPeNQ_400x400.jpeg?1696527447', 'current_price': 617.07, 'market_cap': 4554478084, 'market_cap_rank': 27, 'fully_diluted_valuation': 12958100764, 'total_volume': 305713998, 'high_24h': 671.85, 'low_24h': 601.5, 'price_change_24h': -54.06723446710225, 'price_change_percentage_24h': -8.05608, 'market_cap_change_24h': -334381577.4141588, 'market_cap_change_percentage_24h': -6.83966, 'circulating_supply': 7381023.0, 'total_supply': 21000000.0, 'max_supply': 21000000.0, 'ath': 757.6, 'ath_change_percentage': -18.06424, 'ath_date': '2024-03-07T18:45:36.466Z', 'atl': 30.83, 'atl_change_percentage': 1913.46097, 'atl_date': '2023-05-14T08:57:53.732Z', 'roi': None, 'last_updated': '2024-10-08T00:54:05.434Z'}, {'id': 'aptos', 'symbol': 'apt', 'name': 'Aptos', 'image': 'https://coin-images.coingecko.com/coins/images/26455/large/aptos_round.png?1696525528', 'current_price': 8.91, 'market_cap': 4482021558, 'market_cap_rank': 28, 'fully_diluted_valuation': 9975514711, 'total_volume': 559303098, 'high_24h': 9.29, 'low_24h': 8.46, 'price_change_24h': -0.05442955762588575, 'price_change_percentage_24h': -0.6072, 'market_cap_change_24h': -22231350.747354507, 'market_cap_change_percentage_24h': -0.49356, 'circulating_supply': 503104224.342875, 'total_supply': 1119745526.9904, 'max_supply': None, 'ath': 19.92, 'ath_change_percentage': -55.28954, 'ath_date': '2023-01-26T14:25:17.390Z', 'atl': 3.08, 'atl_change_percentage': 189.19136, 'atl_date': '2022-12-29T21:35:14.796Z', 'roi': None, 'last_updated': '2024-10-08T00:54:04.642Z'}, {'id': 'pepe', 'symbol': 'pepe', 'name': 'Pepe', 'image': 'https://coin-images.coingecko.com/coins/images/29850/large/pepe-token.jpeg?1696528776', 'current_price': 9.89e-06, 'market_cap': 4160848085, 'market_cap_rank': 29, 'fully_diluted_valuation': 4160848085, 'total_volume': 2685682892, 'high_24h': 1.075e-05, 'low_24h': 9.79e-06, 'price_change_24h': -5.62021500895e-07, 'price_change_percentage_24h': -5.37525, 'market_cap_change_24h': -237379054.54402733, 'market_cap_change_percentage_24h': -5.39715, 'circulating_supply': 420690000000000.0, 'total_supply': 420690000000000.0, 'max_supply': 420690000000000.0, 'ath': 1.717e-05, 'ath_change_percentage': -42.2268, 'ath_date': '2024-05-27T08:30:07.709Z', 'atl': 5.5142e-08, 'atl_change_percentage': 17885.15417, 'atl_date': '2023-04-18T02:14:41.591Z', 'roi': None, 'last_updated': '2024-10-08T00:54:02.340Z'}, {'id': 'wrapped-eeth', 'symbol': 'weeth', 'name': 'Wrapped eETH', 'image': 'https://coin-images.coingecko.com/coins/images/33033/large/weETH.png?1701438396', 'current_price': 2554.88, 'market_cap': 4038780433, 'market_cap_rank': 30, 'fully_diluted_valuation': 4038780433, 'total_volume': 30073791, 'high_24h': 2635.83, 'low_24h': 2524.51, 'price_change_24h': -56.44659523926384, 'price_change_percentage_24h': -2.16161, 'market_cap_change_24h': -54462725.86518383, 'market_cap_change_percentage_24h': -1.33055, 'circulating_supply': 1580768.11822637, 'total_supply': 1580768.11822637, 'max_supply': None, 'ath': 4196.87, 'ath_change_percentage': -39.06049, 'ath_date': '2024-03-13T08:29:59.938Z', 'atl': 2231.18, 'atl_change_percentage': 14.62807, 'atl_date': '2024-01-08T03:35:28.624Z', 'roi': None, 'last_updated': '2024-10-08T00:54:12.420Z'}, {'id': 'fetch-ai', 'symbol': 'fet', 'name': 'Artificial Superintelligence Alliance', 'image': 'https://coin-images.coingecko.com/coins/images/5681/large/ASI.png?1719827289', 'current_price': 1.49, 'market_cap': 3876658812, 'market_cap_rank': 31, 'fully_diluted_valuation': 4039354437, 'total_volume': 571576675, 'high_24h': 1.55, 'low_24h': 1.46, 'price_change_24h': -0.02254401432244868, 'price_change_percentage_24h': -1.49514, 'market_cap_change_24h': -57053180.25035906, 'market_cap_change_percentage_24h': -1.45036, 'circulating_supply': 2609959126.672, 'total_supply': 2719493896.672, 'max_supply': 2719493896.672, 'ath': 3.45, 'ath_change_percentage': -57.02791, 'ath_date': '2024-03-28T17:21:18.050Z', 'atl': 0.00816959, 'atl_change_percentage': 18068.83315, 'atl_date': '2020-03-13T02:24:18.347Z', 'roi': {'times': 16.131207571263495, 'currency': 'usd', 'percentage': 1613.1207571263494}, 'last_updated': '2024-10-08T00:54:07.419Z'}, {'id': 'internet-computer', 'symbol': 'icp', 'name': 'Internet Computer', 'image': 'https://coin-images.coingecko.com/coins/images/14495/large/Internet_Computer_logo.png?1696514180', 'current_price': 8.13, 'market_cap': 3837481675, 'market_cap_rank': 32, 'fully_diluted_valuation': 4261162155, 'total_volume': 149290724, 'high_24h': 8.64, 'low_24h': 8.04, 'price_change_24h': -0.4425720487875928, 'price_change_percentage_24h': -5.16321, 'market_cap_change_24h': -205014513.86187172, 'market_cap_change_percentage_24h': -5.07148, 'circulating_supply': 471937376.870267, 'total_supply': 524042030.677131, 'max_supply': None, 'ath': 700.65, 'ath_change_percentage': -98.84109, 'ath_date': '2021-05-10T16:05:53.653Z', 'atl': 2.87, 'atl_change_percentage': 183.414, 'atl_date': '2023-09-22T00:29:57.369Z', 'roi': None, 'last_updated': '2024-10-08T00:54:08.888Z'}, {'id': 'kaspa', 'symbol': 'kas', 'name': 'Kaspa', 'image': 'https://coin-images.coingecko.com/coins/images/25751/large/kaspa-icon-exchanges.png?1696524837', 'current_price': 0.137693, 'market_cap': 3422402884, 'market_cap_rank': 33, 'fully_diluted_valuation': 3422566689, 'total_volume': 49406266, 'high_24h': 0.147201, 'low_24h': 0.135657, 'price_change_24h': -0.006053383405024011, 'price_change_percentage_24h': -4.21116, 'market_cap_change_24h': -175264701.85171556, 'market_cap_change_percentage_24h': -4.87162, 'circulating_supply': 24864690527.4324, 'total_supply': 24865880619.228, 'max_supply': 28704026601.0, 'ath': 0.207411, 'ath_change_percentage': -33.49467, 'ath_date': '2024-08-01T00:40:47.164Z', 'atl': 0.00017105, 'atl_change_percentage': 80542.92002, 'atl_date': '2022-05-26T14:42:59.316Z', 'roi': None, 'last_updated': '2024-10-08T00:54:09.197Z'}, {'id': 'polygon-ecosystem-token', 'symbol': 'pol', 'name': 'POL (ex-MATIC)', 'image': 'https://coin-images.coingecko.com/coins/images/32440/large/polygon.png?1698233684', 'current_price': 0.376926, 'market_cap': 2844275996, 'market_cap_rank': 34, 'fully_diluted_valuation': 3876780009, 'total_volume': 59628192, 'high_24h': 0.389753, 'low_24h': 0.373173, 'price_change_24h': -0.01072502812598436, 'price_change_percentage_24h': -2.76667, 'market_cap_change_24h': -89083731.41555977, 'market_cap_change_percentage_24h': -3.03692, 'circulating_supply': 7536487650.506331, 'total_supply': 10272317000.3188, 'max_supply': None, 'ath': 1.29, 'ath_change_percentage': -70.69669, 'ath_date': '2024-03-13T18:55:06.692Z', 'atl': 0.344976, 'atl_change_percentage': 9.36936, 'atl_date': '2024-08-05T06:45:46.141Z', 'roi': None, 'last_updated': '2024-10-08T00:53:49.166Z'}, {'id': 'ethereum-classic', 'symbol': 'etc', 'name': 'Ethereum Classic', 'image': 'https://coin-images.coingecko.com/coins/images/453/large/ethereum-classic-logo.png?1696501717', 'current_price': 18.7, 'market_cap': 2787253411, 'market_cap_rank': 35, 'fully_diluted_valuation': 3940186706, 'total_volume': 115562256, 'high_24h': 19.1, 'low_24h': 18.54, 'price_change_24h': -0.2404127891239547, 'price_change_percentage_24h': -1.26933, 'market_cap_change_24h': -34460136.58007479, 'market_cap_change_percentage_24h': -1.22125, 'circulating_supply': 149047326.285244, 'total_supply': 210700000.0, 'max_supply': 210700000.0, 'ath': 167.09, 'ath_change_percentage': -88.80786, 'ath_date': '2021-05-06T18:34:22.133Z', 'atl': 0.615038, 'atl_change_percentage': 2940.53103, 'atl_date': '2016-07-25T00:00:00.000Z', 'roi': {'times': 40.55485369483518, 'currency': 'usd', 'percentage': 4055.485369483518}, 'last_updated': '2024-10-08T00:54:08.346Z'}, {'id': 'stellar', 'symbol': 'xlm', 'name': 'Stellar', 'image': 'https://coin-images.coingecko.com/coins/images/100/large/Stellar_symbol_black_RGB.png?1696501482', 'current_price': 0.091505, 'market_cap': 2718689271, 'market_cap_rank': 36, 'fully_diluted_valuation': 4575830767, 'total_volume': 55794210, 'high_24h': 0.093297, 'low_24h': 0.090686, 'price_change_24h': -0.001792341838620773, 'price_change_percentage_24h': -1.92111, 'market_cap_change_24h': -51736126.038962364, 'market_cap_change_percentage_24h': -1.86744, 'circulating_supply': 29708118282.3443, 'total_supply': 50001786931.8027, 'max_supply': 50001786931.8027, 'ath': 0.875563, 'ath_change_percentage': -89.54997, 'ath_date': '2018-01-03T00:00:00.000Z', 'atl': 0.00047612, 'atl_change_percentage': 19116.98588, 'atl_date': '2015-03-05T00:00:00.000Z', 'roi': None, 'last_updated': '2024-10-08T00:54:11.358Z'}, {'id': 'monero', 'symbol': 'xmr', 'name': 'Monero', 'image': 'https://coin-images.coingecko.com/coins/images/69/large/monero_logo.png?1696501460', 'current_price': 144.06, 'market_cap': 2658495109, 'market_cap_rank': 37, 'fully_diluted_valuation': 2658495109, 'total_volume': 49881391, 'high_24h': 148.72, 'low_24h': 143.98, 'price_change_24h': -4.402376663747191, 'price_change_percentage_24h': -2.96526, 'market_cap_change_24h': -79142990.84689522, 'market_cap_change_percentage_24h': -2.89092, 'circulating_supply': 18446744.0737096, 'total_supply': 18446744.0737096, 'max_supply': None, 'ath': 542.33, 'ath_change_percentage': -73.40115, 'ath_date': '2018-01-09T00:00:00.000Z', 'atl': 0.216177, 'atl_change_percentage': 66628.90964, 'atl_date': '2015-01-14T00:00:00.000Z', 'roi': None, 'last_updated': '2024-10-08T00:54:12.179Z'}, {'id': 'blockstack', 'symbol': 'stx', 'name': 'Stacks', 'image': 'https://coin-images.coingecko.com/coins/images/2069/large/Stacks_Logo_png.png?1709979332', 'current_price': 1.77, 'market_cap': 2636405645, 'market_cap_rank': 38, 'fully_diluted_valuation': 2636405645, 'total_volume': 148650701, 'high_24h': 1.88, 'low_24h': 1.76, 'price_change_24h': -0.10597245605099315, 'price_change_percentage_24h': -5.65908, 'market_cap_change_24h': -157654404.66367483, 'market_cap_change_percentage_24h': -5.64248, 'circulating_supply': 1492329684.93845, 'total_supply': 1492329684.93845, 'max_supply': 1818000000.0, 'ath': 3.86, 'ath_change_percentage': -54.22821, 'ath_date': '2024-04-01T12:34:58.342Z', 'atl': 0.04559639, 'atl_change_percentage': 3779.18767, 'atl_date': '2020-03-13T02:29:26.415Z', 'roi': {'times': 13.721979508556592, 'currency': 'usd', 'percentage': 1372.1979508556592}, 'last_updated': '2024-10-08T00:54:05.953Z'}, {'id': 'first-digital-usd', 'symbol': 'fdusd', 'name': 'First Digital USD', 'image': 'https://coin-images.coingecko.com/coins/images/31079/large/firstfigital.jpeg?1696529912', 'current_price': 0.999501, 'market_cap': 2614879689, 'market_cap_rank': 39, 'fully_diluted_valuation': 2614879689, 'total_volume': 5277645305, 'high_24h': 1.009, 'low_24h': 0.989271, 'price_change_24h': -0.002162259148526635, 'price_change_percentage_24h': -0.21587, 'market_cap_change_24h': -2509489.620900631, 'market_cap_change_percentage_24h': -0.09588, 'circulating_supply': 2616235583.37, 'total_supply': 2616235583.37, 'max_supply': None, 'ath': 1.089, 'ath_change_percentage': -8.09515, 'ath_date': '2024-05-20T19:42:15.377Z', 'atl': 0.942129, 'atl_change_percentage': 6.18864, 'atl_date': '2023-08-17T21:55:41.478Z', 'roi': None, 'last_updated': '2024-10-08T00:54:07.584Z'}, {'id': 'dogwifcoin', 'symbol': 'wif', 'name': 'dogwifhat', 'image': 'https://coin-images.coingecko.com/coins/images/33566/large/dogwifhat.jpg?1702499428', 'current_price': 2.56, 'market_cap': 2553931562, 'market_cap_rank': 40, 'fully_diluted_valuation': 2553931562, 'total_volume': 1577781813, 'high_24h': 2.77, 'low_24h': 2.52, 'price_change_24h': -0.07085253704175454, 'price_change_percentage_24h': -2.69691, 'market_cap_change_24h': -69753420.44635868, 'market_cap_change_percentage_24h': -2.65861, 'circulating_supply': 998926392.0, 'total_supply': 998926392.0, 'max_supply': 998926392.0, 'ath': 4.83, 'ath_change_percentage': -47.0231, 'ath_date': '2024-03-31T09:34:58.366Z', 'atl': 0.00155464, 'atl_change_percentage': 164335.54227, 'atl_date': '2023-12-13T07:13:50.873Z', 'roi': None, 'last_updated': '2024-10-08T00:54:06.464Z'}, {'id': 'okb', 'symbol': 'okb', 'name': 'OKB', 'image': 'https://coin-images.coingecko.com/coins/images/4463/large/WeChat_Image_20220118095654.png?1696505053', 'current_price': 41.75, 'market_cap': 2504875206, 'market_cap_rank': 41, 'fully_diluted_valuation': 9850742591, 'total_volume': 3021964, 'high_24h': 42.23, 'low_24h': 41.49, 'price_change_24h': 0.133862, 'price_change_percentage_24h': 0.32167, 'market_cap_change_24h': 9021972, 'market_cap_change_percentage_24h': 0.36148, 'circulating_supply': 60000000.0, 'total_supply': 235957685.3, 'max_supply': 300000000.0, 'ath': 73.8, 'ath_change_percentage': -43.40078, 'ath_date': '2024-03-14T00:30:12.502Z', 'atl': 0.580608, 'atl_change_percentage': 7094.54711, 'atl_date': '2019-01-14T00:00:00.000Z', 'roi': None, 'last_updated': '2024-10-08T00:54:11.193Z'}, {'id': 'ethena-usde', 'symbol': 'usde', 'name': 'Ethena USDe', 'image': 'https://coin-images.coingecko.com/coins/images/33613/large/USDE.png?1716355685', 'current_price': 0.998868, 'market_cap': 2475501311, 'market_cap_rank': 42, 'fully_diluted_valuation': 2475501311, 'total_volume': 42239822, 'high_24h': 1.001, 'low_24h': 0.99399, 'price_change_24h': -0.000324026932799759, 'price_change_percentage_24h': -0.03243, 'market_cap_change_24h': -735411.9714083672, 'market_cap_change_percentage_24h': -0.0297, 'circulating_supply': 2478118745.28326, 'total_supply': 2478118745.28326, 'max_supply': None, 'ath': 1.032, 'ath_change_percentage': -3.18123, 'ath_date': '2023-12-20T15:38:34.596Z', 'atl': 0.929486, 'atl_change_percentage': 7.50557, 'atl_date': '2024-10-04T07:57:15.809Z', 'roi': None, 'last_updated': '2024-10-08T00:54:08.938Z'}, {'id': 'immutable-x', 'symbol': 'imx', 'name': 'Immutable', 'image': 'https://coin-images.coingecko.com/coins/images/17233/large/immutableX-symbol-BLK-RGB.png?1696516787', 'current_price': 1.49, 'market_cap': 2399110937, 'market_cap_rank': 43, 'fully_diluted_valuation': 2988988709, 'total_volume': 98646439, 'high_24h': 1.58, 'low_24h': 1.48, 'price_change_24h': -0.04880323823546994, 'price_change_percentage_24h': -3.16226, 'market_cap_change_24h': -77613561.05947065, 'market_cap_change_percentage_24h': -3.13372, 'circulating_supply': 1605299431.3898141, 'total_supply': 2000000000.0, 'max_supply': 2000000000.0, 'ath': 9.52, 'ath_change_percentage': -84.26299, 'ath_date': '2021-11-26T01:03:01.536Z', 'atl': 0.378055, 'atl_change_percentage': 296.27954, 'atl_date': '2022-12-31T07:36:37.649Z', 'roi': None, 'last_updated': '2024-10-08T00:54:08.984Z'}, {'id': 'filecoin', 'symbol': 'fil', 'name': 'Filecoin', 'image': 'https://coin-images.coingecko.com/coins/images/12817/large/filecoin.png?1696512609', 'current_price': 3.74, 'market_cap': 2212048994, 'market_cap_rank': 44, 'fully_diluted_valuation': 7333073696, 'total_volume': 220501731, 'high_24h': 3.81, 'low_24h': 3.69, 'price_change_24h': 0.00388424, 'price_change_percentage_24h': 0.10391, 'market_cap_change_24h': 3262126, 'market_cap_change_percentage_24h': 0.14769, 'circulating_supply': 591219863.0, 'total_supply': 1959928934.0, 'max_supply': 1959928934.0, 'ath': 236.84, 'ath_change_percentage': -98.42174, 'ath_date': '2021-04-01T13:29:41.564Z', 'atl': 2.64, 'atl_change_percentage': 41.53757, 'atl_date': '2022-12-16T22:45:28.552Z', 'roi': None, 'last_updated': '2024-10-08T00:54:07.874Z'}, {'id': 'aave', 'symbol': 'aave', 'name': 'Aave', 'image': 'https://coin-images.coingecko.com/coins/images/12645/large/aave-token-round.png?1720472354', 'current_price': 146.68, 'market_cap': 2192912572, 'market_cap_rank': 45, 'fully_diluted_valuation': 2346687419, 'total_volume': 317227189, 'high_24h': 154.37, 'low_24h': 145.63, 'price_change_24h': -5.858279094344567, 'price_change_percentage_24h': -3.84065, 'market_cap_change_24h': -87181126.71949911, 'market_cap_change_percentage_24h': -3.82358, 'circulating_supply': 14951544.4115039, 'total_supply': 16000000.0, 'max_supply': 16000000.0, 'ath': 661.69, 'ath_change_percentage': -77.76392, 'ath_date': '2021-05-18T21:19:59.514Z', 'atl': 26.02, 'atl_change_percentage': 465.39646, 'atl_date': '2020-11-05T09:20:11.928Z', 'roi': None, 'last_updated': '2024-10-08T00:54:13.358Z'}, {'id': 'crypto-com-chain', 'symbol': 'cro', 'name': 'Cronos', 'image': 'https://coin-images.coingecko.com/coins/images/7310/large/cro_token_logo.png?1696507599', 'current_price': 0.078844, 'market_cap': 2129405535, 'market_cap_rank': 46, 'fully_diluted_valuation': 2364898273, 'total_volume': 5713307, 'high_24h': 0.081471, 'low_24h': 0.078325, 'price_change_24h': -0.002135162614234229, 'price_change_percentage_24h': -2.63669, 'market_cap_change_24h': -60340163.39772034, 'market_cap_change_percentage_24h': -2.75558, 'circulating_supply': 27012648607.4253, 'total_supply': 30000000000.0, 'max_supply': None, 'ath': 0.965407, 'ath_change_percentage': -91.82827, 'ath_date': '2021-11-24T15:53:54.855Z', 'atl': 0.0121196, 'atl_change_percentage': 550.93336, 'atl_date': '2019-02-08T00:00:00.000Z', 'roi': None, 'last_updated': '2024-10-08T00:54:07.102Z'}, {'id': 'optimism', 'symbol': 'op', 'name': 'Optimism', 'image': 'https://coin-images.coingecko.com/coins/images/25244/large/Optimism.png?1696524385', 'current_price': 1.67, 'market_cap': 2101136824, 'market_cap_rank': 47, 'fully_diluted_valuation': 7190284537, 'total_volume': 218428886, 'high_24h': 1.73, 'low_24h': 1.64, 'price_change_24h': -0.03476149712388876, 'price_change_percentage_24h': -2.03669, 'market_cap_change_24h': -39040505.690792084, 'market_cap_change_percentage_24h': -1.82417, 'circulating_supply': 1255070491.0, 'total_supply': 4294967296.0, 'max_supply': 4294967296.0, 'ath': 4.84, 'ath_change_percentage': -65.39861, 'ath_date': '2024-03-06T08:35:50.817Z', 'atl': 0.402159, 'atl_change_percentage': 316.79983, 'atl_date': '2022-06-18T20:54:52.178Z', 'roi': None, 'last_updated': '2024-10-08T00:54:10.229Z'}, {'id': 'render-token', 'symbol': 'render', 'name': 'Render', 'image': 'https://coin-images.coingecko.com/coins/images/11636/large/rndr.png?1696511529', 'current_price': 5.32, 'market_cap': 2086965284, 'market_cap_rank': 48, 'fully_diluted_valuation': 2830162803, 'total_volume': 189902209, 'high_24h': 5.66, 'low_24h': 5.25, 'price_change_24h': -0.23595866218748007, 'price_change_percentage_24h': -4.24782, 'market_cap_change_24h': -90450600.43532825, 'market_cap_change_percentage_24h': -4.15403, 'circulating_supply': 392459381.455492, 'total_supply': 532219654.735492, 'max_supply': None, 'ath': 13.53, 'ath_change_percentage': -60.69465, 'ath_date': '2024-03-17T16:30:24.636Z', 'atl': 0.03665669, 'atl_change_percentage': 14412.65249, 'atl_date': '2020-06-16T13:22:25.900Z', 'roi': None, 'last_updated': '2024-10-08T00:54:10.739Z'}, {'id': 'injective-protocol', 'symbol': 'inj', 'name': 'Injective', 'image': 'https://coin-images.coingecko.com/coins/images/12882/large/Secondary_Symbol.png?1696512670', 'current_price': 20.63, 'market_cap': 2020107435, 'market_cap_rank': 49, 'fully_diluted_valuation': 2067087743, 'total_volume': 162234744, 'high_24h': 21.68, 'low_24h': 20.41, 'price_change_24h': -0.5420461942348993, 'price_change_percentage_24h': -2.56032, 'market_cap_change_24h': -38736183.09372449, 'market_cap_change_percentage_24h': -1.88145, 'circulating_supply': 97727222.33, 'total_supply': 100000000.0, 'max_supply': None, 'ath': 52.62, 'ath_change_percentage': -60.76848, 'ath_date': '2024-03-14T15:06:22.124Z', 'atl': 0.657401, 'atl_change_percentage': 3040.22836, 'atl_date': '2020-11-03T16:19:30.576Z', 'roi': None, 'last_updated': '2024-10-08T00:54:08.301Z'}, {'id': 'arbitrum', 'symbol': 'arb', 'name': 'Arbitrum', 'image': 'https://coin-images.coingecko.com/coins/images/16547/large/arb.jpg?1721358242', 'current_price': 0.55172, 'market_cap': 1995610038, 'market_cap_rank': 50, 'fully_diluted_valuation': 5517172560, 'total_volume': 305793045, 'high_24h': 0.574299, 'low_24h': 0.549433, 'price_change_24h': -0.01640000679415732, 'price_change_percentage_24h': -2.88672, 'market_cap_change_24h': -58973496.59108758, 'market_cap_change_percentage_24h': -2.87034, 'circulating_supply': 3617088312.0, 'total_supply': 10000000000.0, 'max_supply': 10000000000.0, 'ath': 2.39, 'ath_change_percentage': -76.88637, 'ath_date': '2024-01-12T12:29:59.231Z', 'atl': 0.431578, 'atl_change_percentage': 28.01435, 'atl_date': '2024-08-05T11:35:54.024Z', 'roi': None, 'last_updated': '2024-10-08T00:54:12.965Z'}, {'id': 'hedera-hashgraph', 'symbol': 'hbar', 'name': 'Hedera', 'image': 'https://coin-images.coingecko.com/coins/images/3688/large/hbar.png?1696504364', 'current_price': 0.052714, 'market_cap': 1984767944, 'market_cap_rank': 51, 'fully_diluted_valuation': 2635626384, 'total_volume': 58643085, 'high_24h': 0.055465, 'low_24h': 0.052442, 'price_change_24h': -0.002289720403595014, 'price_change_percentage_24h': -4.16286, 'market_cap_change_24h': -87166884.84962511, 'market_cap_change_percentage_24h': -4.20703, 'circulating_supply': 37652680130.7546, 'total_supply': 50000000000.0, 'max_supply': 50000000000.0, 'ath': 0.569229, 'ath_change_percentage': -90.73263, 'ath_date': '2021-09-15T10:40:28.318Z', 'atl': 0.00986111, 'atl_change_percentage': 434.95523, 'atl_date': '2020-01-02T17:30:24.852Z', 'roi': None, 'last_updated': '2024-10-08T00:54:07.816Z'}, {'id': 'mantle', 'symbol': 'mnt', 'name': 'Mantle', 'image': 'https://coin-images.coingecko.com/coins/images/30980/large/token-logo.png?1696529819', 'current_price': 0.594723, 'market_cap': 1942595143, 'market_cap_rank': 52, 'fully_diluted_valuation': 3698255282, 'total_volume': 83557233, 'high_24h': 0.617403, 'low_24h': 0.590237, 'price_change_24h': -0.020384026293793656, 'price_change_percentage_24h': -3.3139, 'market_cap_change_24h': -65637588.91737676, 'market_cap_change_percentage_24h': -3.26843, 'circulating_supply': 3266841707.83684, 'total_supply': 6219316794.99, 'max_supply': 6219316794.99, 'ath': 1.54, 'ath_change_percentage': -61.31909, 'ath_date': '2024-04-08T09:45:25.482Z', 'atl': 0.307978, 'atl_change_percentage': 93.25706, 'atl_date': '2023-10-18T02:50:46.942Z', 'roi': None, 'last_updated': '2024-10-08T00:54:08.666Z'}, {'id': 'fantom', 'symbol': 'ftm', 'name': 'Fantom', 'image': 'https://coin-images.coingecko.com/coins/images/4001/large/Fantom_round.png?1696504642', 'current_price': 0.680296, 'market_cap': 1904364725, 'market_cap_rank': 53, 'fully_diluted_valuation': 2156613951, 'total_volume': 442766255, 'high_24h': 0.692213, 'low_24h': 0.643663, 'price_change_24h': 0.02108022, 'price_change_percentage_24h': 3.19777, 'market_cap_change_24h': 51058008, 'market_cap_change_percentage_24h': 2.75497, 'circulating_supply': 2803634835.52659, 'total_supply': 3175000000.0, 'max_supply': 3175000000.0, 'ath': 3.46, 'ath_change_percentage': -80.42443, 'ath_date': '2021-10-28T05:19:39.845Z', 'atl': 0.00190227, 'atl_change_percentage': 35490.93498, 'atl_date': '2020-03-13T02:25:38.280Z', 'roi': {'times': 21.676526443109893, 'currency': 'usd', 'percentage': 2167.652644310989}, 'last_updated': '2024-10-08T00:54:08.083Z'}, {'id': 'vechain', 'symbol': 'vet', 'name': 'VeChain', 'image': 'https://coin-images.coingecko.com/coins/images/1167/large/VET_Token_Icon.png?1710013505', 'current_price': 0.02305745, 'market_cap': 1866097633, 'market_cap_rank': 54, 'fully_diluted_valuation': 1981310122, 'total_volume': 33029041, 'high_24h': 0.02413624, 'low_24h': 0.02287468, 'price_change_24h': -0.000579121553516551, 'price_change_percentage_24h': -2.45011, 'market_cap_change_24h': -48530189.73806381, 'market_cap_change_percentage_24h': -2.53471, 'circulating_supply': 80985041177.0, 'total_supply': 85985041177.0, 'max_supply': 86712634466.0, 'ath': 0.280991, 'ath_change_percentage': -91.78701, 'ath_date': '2021-04-19T01:08:21.675Z', 'atl': 0.00191713, 'atl_change_percentage': 1103.76506, 'atl_date': '2020-03-13T02:29:59.652Z', 'roi': {'times': 2.315297764204298, 'currency': 'eth', 'percentage': 231.52977642042976}, 'last_updated': '2024-10-08T00:54:04.449Z'}, {'id': 'cosmos', 'symbol': 'atom', 'name': 'Cosmos Hub', 'image': 'https://coin-images.coingecko.com/coins/images/1481/large/cosmos_hub.png?1696502525', 'current_price': 4.44, 'market_cap': 1735226482, 'market_cap_rank': 55, 'fully_diluted_valuation': 1736299828, 'total_volume': 157094956, 'high_24h': 4.78, 'low_24h': 4.42, 'price_change_24h': -0.2745205030004252, 'price_change_percentage_24h': -5.82303, 'market_cap_change_24h': -106653058.32591224, 'market_cap_change_percentage_24h': -5.79045, 'circulating_supply': 390688369.813272, 'total_supply': 390930035.085365, 'max_supply': None, 'ath': 44.45, 'ath_change_percentage': -90.00078, 'ath_date': '2022-01-17T00:34:41.497Z', 'atl': 1.16, 'atl_change_percentage': 283.12318, 'atl_date': '2020-03-13T02:27:44.591Z', 'roi': {'times': 43.39870942426602, 'currency': 'usd', 'percentage': 4339.870942426602}, 'last_updated': '2024-10-08T00:54:06.660Z'}, {'id': 'thorchain', 'symbol': 'rune', 'name': 'THORChain', 'image': 'https://coin-images.coingecko.com/coins/images/6595/large/Rune200x200.png?1696506946', 'current_price': 5.09, 'market_cap': 1713439020, 'market_cap_rank': 56, 'fully_diluted_valuation': 2107376729, 'total_volume': 436029070, 'high_24h': 5.32, 'low_24h': 4.99, 'price_change_24h': 0.092116, 'price_change_percentage_24h': 1.8445, 'market_cap_change_24h': 30384714, 'market_cap_change_percentage_24h': 1.80533, 'circulating_supply': 336760873.0, 'total_supply': 414185751.0, 'max_supply': 500000000.0, 'ath': 20.87, 'ath_change_percentage': -75.60622, 'ath_date': '2021-05-19T00:30:09.436Z', 'atl': 0.00851264, 'atl_change_percentage': 59698.01566, 'atl_date': '2019-09-28T00:00:00.000Z', 'roi': {'times': 132.84719235436123, 'currency': 'usd', 'percentage': 13284.719235436123}, 'last_updated': '2024-10-08T00:54:11.506Z'}, {'id': 'whitebit', 'symbol': 'wbt', 'name': 'WhiteBIT Coin', 'image': 'https://coin-images.coingecko.com/coins/images/27045/large/wbt_token.png?1696526096', 'current_price': 11.61, 'market_cap': 1672767757, 'market_cap_rank': 57, 'fully_diluted_valuation': 3986192957, 'total_volume': 5269580, 'high_24h': 11.74, 'low_24h': 11.45, 'price_change_24h': 0.054923, 'price_change_percentage_24h': 0.47536, 'market_cap_change_24h': 8356197, 'market_cap_change_percentage_24h': 0.50205, 'circulating_supply': 144118517.10815412, 'total_supply': 343433340.0, 'max_supply': 400000000.0, 'ath': 14.64, 'ath_change_percentage': -20.66611, 'ath_date': '2022-10-28T12:32:18.119Z', 'atl': 3.06, 'atl_change_percentage': 279.46514, 'atl_date': '2023-02-13T19:01:21.899Z', 'roi': None, 'last_updated': '2024-10-08T00:54:12.576Z'}, {'id': 'the-graph', 'symbol': 'grt', 'name': 'The Graph', 'image': 'https://coin-images.coingecko.com/coins/images/13397/large/Graph_Token.png?1696513159', 'current_price': 0.16643, 'market_cap': 1590930683, 'market_cap_rank': 58, 'fully_diluted_valuation': 1797445718, 'total_volume': 74994229, 'high_24h': 0.175174, 'low_24h': 0.164311, 'price_change_24h': -0.002237407739360153, 'price_change_percentage_24h': -1.32652, 'market_cap_change_24h': -19839233.915035248, 'market_cap_change_percentage_24h': -1.23166, 'circulating_supply': 9548531509.16547, 'total_supply': 10788004319.0, 'max_supply': 10788004319.0, 'ath': 2.84, 'ath_change_percentage': -94.13502, 'ath_date': '2021-02-12T07:28:45.775Z', 'atl': 0.052051, 'atl_change_percentage': 220.17379, 'atl_date': '2022-11-22T10:05:03.503Z', 'roi': None, 'last_updated': '2024-10-08T00:54:11.707Z'}, {'id': 'sei-network', 'symbol': 'sei', 'name': 'Sei', 'image': 'https://coin-images.coingecko.com/coins/images/28205/large/Sei_Logo_-_Transparent.png?1696527207', 'current_price': 0.436364, 'market_cap': 1537777839, 'market_cap_rank': 59, 'fully_diluted_valuation': 4362662218, 'total_volume': 385107557, 'high_24h': 0.45975, 'low_24h': 0.425647, 'price_change_24h': -0.012570966005509167, 'price_change_percentage_24h': -2.80018, 'market_cap_change_24h': -44875622.576061964, 'market_cap_change_percentage_24h': -2.83547, 'circulating_supply': 3524861111.0, 'total_supply': 10000000000.0, 'max_supply': None, 'ath': 1.14, 'ath_change_percentage': -61.71689, 'ath_date': '2024-03-16T02:30:23.904Z', 'atl': 0.095364, 'atl_change_percentage': 357.53176, 'atl_date': '2023-10-19T08:05:30.655Z', 'roi': None, 'last_updated': '2024-10-08T00:54:11.382Z'}, {'id': 'bitget-token', 'symbol': 'bgb', 'name': 'Bitget Token', 'image': 'https://coin-images.coingecko.com/coins/images/11610/large/icon_colour.png?1696511504', 'current_price': 1.075, 'market_cap': 1505112149, 'market_cap_rank': 60, 'fully_diluted_valuation': 2150158677, 'total_volume': 4809367, 'high_24h': 1.14, 'low_24h': 0.641602, 'price_change_24h': -0.029675512038628504, 'price_change_percentage_24h': -2.68607, 'market_cap_change_24h': -41599600.1858089, 'market_cap_change_percentage_24h': -2.68955, 'circulating_supply': 1400001000.0, 'total_supply': 2000000000.0, 'max_supply': 2000000000.0, 'ath': 1.48, 'ath_change_percentage': -27.18174, 'ath_date': '2024-06-01T03:50:55.951Z', 'atl': 0.0142795, 'atl_change_percentage': 7443.46905, 'atl_date': '2020-06-25T04:17:05.895Z', 'roi': None, 'last_updated': '2024-10-08T00:54:05.579Z'}, {'id': 'bonk', 'symbol': 'bonk', 'name': 'Bonk', 'image': 'https://coin-images.coingecko.com/coins/images/28600/large/bonk.jpg?1696527587', 'current_price': 2.134e-05, 'market_cap': 1482766707, 'market_cap_rank': 61, 'fully_diluted_valuation': 1996093347, 'total_volume': 418331264, 'high_24h': 2.327e-05, 'low_24h': 2.116e-05, 'price_change_24h': -1.444364956781e-06, 'price_change_percentage_24h': -6.33855, 'market_cap_change_24h': -100689387.01500964, 'market_cap_change_percentage_24h': -6.35884, 'circulating_supply': 69474461693558.484, 'total_supply': 93526183276778.0, 'max_supply': 93526183276778.0, 'ath': 4.547e-05, 'ath_change_percentage': -53.06035, 'ath_date': '2024-03-04T17:05:29.594Z', 'atl': 8.6142e-08, 'atl_change_percentage': 24677.40406, 'atl_date': '2022-12-29T22:48:46.755Z', 'roi': None, 'last_updated': '2024-10-08T00:54:06.906Z'}, {'id': 'binance-peg-weth', 'symbol': 'weth', 'name': 'Binance-Peg WETH', 'image': 'https://coin-images.coingecko.com/coins/images/39580/large/weth.png?1723006716', 'current_price': 2434.55, 'market_cap': 1472855920, 'market_cap_rank': 62, 'fully_diluted_valuation': 1472855920, 'total_volume': 52219432, 'high_24h': 2512.03, 'low_24h': 2423.7, 'price_change_24h': -52.02834219076749, 'price_change_percentage_24h': -2.09236, 'market_cap_change_24h': -32065616.701003075, 'market_cap_change_percentage_24h': -2.13072, 'circulating_supply': 604999.999959841, 'total_supply': 604999.999959841, 'max_supply': None, 'ath': 2808.56, 'ath_change_percentage': -13.40018, 'ath_date': '2024-08-24T16:52:46.091Z', 'atl': 2171.8, 'atl_change_percentage': 11.99047, 'atl_date': '2024-09-06T21:02:52.910Z', 'roi': None, 'last_updated': '2024-10-08T00:54:04.960Z'}, {'id': 'floki', 'symbol': 'floki', 'name': 'FLOKI', 'image': 'https://coin-images.coingecko.com/coins/images/16746/large/PNG_image.png?1696516318', 'current_price': 0.00013831, 'market_cap': 1340262428, 'market_cap_rank': 63, 'fully_diluted_valuation': 1383218927, 'total_volume': 371506712, 'high_24h': 0.00014615, 'low_24h': 0.00013686, 'price_change_24h': -5.493776205175e-06, 'price_change_percentage_24h': -3.82021, 'market_cap_change_24h': -53932127.53149462, 'market_cap_change_percentage_24h': -3.86834, 'circulating_supply': 9689445405951.0, 'total_supply': 10000000000000.0, 'max_supply': 10000000000000.0, 'ath': 0.00034495, 'ath_change_percentage': -59.87297, 'ath_date': '2024-06-05T07:25:59.137Z', 'atl': 8.428e-08, 'atl_change_percentage': 164136.20747, 'atl_date': '2021-07-06T01:11:20.438Z', 'roi': None, 'last_updated': '2024-10-08T00:54:07.994Z'}, {'id': 'rocket-pool-eth', 'symbol': 'reth', 'name': 'Rocket Pool ETH', 'image': 'https://coin-images.coingecko.com/coins/images/20764/large/reth.png?1696520159', 'current_price': 2719.44, 'market_cap': 1328506348, 'market_cap_rank': 64, 'fully_diluted_valuation': 1328506348, 'total_volume': 5214534, 'high_24h': 2808.84, 'low_24h': 2696.73, 'price_change_24h': -64.3257142784546, 'price_change_percentage_24h': -2.31075, 'market_cap_change_24h': -30626102.881741285, 'market_cap_change_percentage_24h': -2.25336, 'circulating_supply': 488193.944227492, 'total_supply': 488193.944227492, 'max_supply': None, 'ath': 4814.31, 'ath_change_percentage': -43.50101, 'ath_date': '2021-12-01T08:03:50.749Z', 'atl': 887.26, 'atl_change_percentage': 206.56468, 'atl_date': '2022-06-18T20:55:45.957Z', 'roi': None, 'last_updated': '2024-10-08T00:54:10.671Z'}, {'id': 'theta-token', 'symbol': 'theta', 'name': 'Theta Network', 'image': 'https://coin-images.coingecko.com/coins/images/2538/large/theta-token-logo.png?1696503349', 'current_price': 1.31, 'market_cap': 1305909326, 'market_cap_rank': 65, 'fully_diluted_valuation': 1305909326, 'total_volume': 19702810, 'high_24h': 1.37, 'low_24h': 1.3, 'price_change_24h': -0.05468473696731757, 'price_change_percentage_24h': -4.02037, 'market_cap_change_24h': -52673402.857219934, 'market_cap_change_percentage_24h': -3.87708, 'circulating_supply': 1000000000.0, 'total_supply': 1000000000.0, 'max_supply': 1000000000.0, 'ath': 15.72, 'ath_change_percentage': -91.68442, 'ath_date': '2021-04-16T13:15:11.190Z', 'atl': 0.04039979, 'atl_change_percentage': 3135.62621, 'atl_date': '2020-03-13T02:24:16.483Z', 'roi': {'times': 7.7033697367491865, 'currency': 'usd', 'percentage': 770.3369736749187}, 'last_updated': '2024-10-08T00:54:11.319Z'}, {'id': 'popcat', 'symbol': 'popcat', 'name': 'Popcat', 'image': 'https://coin-images.coingecko.com/coins/images/33760/large/image.jpg?1702964227', 'current_price': 1.28, 'market_cap': 1254180237, 'market_cap_rank': 66, 'fully_diluted_valuation': 1254180237, 'total_volume': 166807604, 'high_24h': 1.47, 'low_24h': 1.27, 'price_change_24h': -0.1536987502902194, 'price_change_percentage_24h': -10.72276, 'market_cap_change_24h': -149282754.86362696, 'market_cap_change_percentage_24h': -10.63674, 'circulating_supply': 979978669.96, 'total_supply': 979978669.96, 'max_supply': 979978694.0, 'ath': 1.47, 'ath_change_percentage': -13.18589, 'ath_date': '2024-10-07T08:39:47.267Z', 'atl': 0.00379728, 'atl_change_percentage': 33597.19423, 'atl_date': '2024-01-05T15:34:24.842Z', 'roi': None, 'last_updated': '2024-10-08T00:53:24.463Z'}, {'id': 'arweave', 'symbol': 'ar', 'name': 'Arweave', 'image': 'https://coin-images.coingecko.com/coins/images/4343/large/oRt6SiEN_400x400.jpg?1696504946', 'current_price': 19.03, 'market_cap': 1245444184, 'market_cap_rank': 67, 'fully_diluted_valuation': 1245444184, 'total_volume': 93459497, 'high_24h': 20.35, 'low_24h': 18.83, 'price_change_24h': -1.102395992722606, 'price_change_percentage_24h': -5.47499, 'market_cap_change_24h': -72086752.11991191, 'market_cap_change_percentage_24h': -5.47135, 'circulating_supply': 65454185.5381511, 'total_supply': 65454185.5381511, 'max_supply': 66000000.0, 'ath': 89.24, 'ath_change_percentage': -78.66006, 'ath_date': '2021-11-05T04:14:42.689Z', 'atl': 0.298788, 'atl_change_percentage': 6273.32719, 'atl_date': '2020-01-31T06:47:36.543Z', 'roi': {'times': 24.719889503069542, 'currency': 'usd', 'percentage': 2471.988950306954}, 'last_updated': '2024-10-08T00:54:04.701Z'}, {'id': 'maker', 'symbol': 'mkr', 'name': 'Maker', 'image': 'https://coin-images.coingecko.com/coins/images/1364/large/Mark_Maker.png?1696502423', 'current_price': 1406.89, 'market_cap': 1228618819, 'market_cap_rank': 68, 'fully_diluted_valuation': 1271387721, 'total_volume': 141868687, 'high_24h': 1484.9, 'low_24h': 1388.68, 'price_change_24h': -65.40749774075653, 'price_change_percentage_24h': -4.44254, 'market_cap_change_24h': -63091679.83813453, 'market_cap_change_percentage_24h': -4.88435, 'circulating_supply': 874256.939689837, 'total_supply': 904690.308012115, 'max_supply': 1005577.0, 'ath': 6292.31, 'ath_change_percentage': -77.6293, 'ath_date': '2021-05-03T21:54:29.333Z', 'atl': 168.36, 'atl_change_percentage': 736.09842, 'atl_date': '2020-03-16T20:52:36.527Z', 'roi': None, 'last_updated': '2024-10-08T00:54:10.987Z'}, {'id': 'mantle-staked-ether', 'symbol': 'meth', 'name': 'Mantle Staked Ether', 'image': 'https://coin-images.coingecko.com/coins/images/33345/large/symbol_transparent_bg.png?1701697066', 'current_price': 2540.6, 'market_cap': 1203906007, 'market_cap_rank': 69, 'fully_diluted_valuation': 1203906007, 'total_volume': 46913247, 'high_24h': 2625.66, 'low_24h': 2510.44, 'price_change_24h': -54.040076595622395, 'price_change_percentage_24h': -2.08276, 'market_cap_change_24h': -28273549.475244284, 'market_cap_change_percentage_24h': -2.2946, 'circulating_supply': 474094.100298458, 'total_supply': 474094.100298458, 'max_supply': None, 'ath': 4729.53, 'ath_change_percentage': -46.19013, 'ath_date': '2024-03-27T05:26:27.333Z', 'atl': 2142.02, 'atl_change_percentage': 18.81108, 'atl_date': '2023-12-18T10:41:32.686Z', 'roi': None, 'last_updated': '2024-10-08T00:54:10.632Z'}, {'id': 'mantra-dao', 'symbol': 'om', 'name': 'MANTRA', 'image': 'https://coin-images.coingecko.com/coins/images/12151/large/OM_Token.png?1696511991', 'current_price': 1.4, 'market_cap': 1192208346, 'market_cap_rank': 70, 'fully_diluted_valuation': 1246554201, 'total_volume': 55717062, 'high_24h': 1.47, 'low_24h': 1.38, 'price_change_24h': -0.008372729468661968, 'price_change_percentage_24h': -0.59314, 'market_cap_change_24h': 993214, 'market_cap_change_percentage_24h': 0.08338, 'circulating_supply': 850136119.15, 'total_supply': 888888888.0, 'max_supply': 888888888.0, 'ath': 1.47, 'ath_change_percentage': -4.68623, 'ath_date': '2024-10-07T01:10:47.695Z', 'atl': 0.01726188, 'atl_change_percentage': 8009.94779, 'atl_date': '2023-10-12T17:25:09.068Z', 'roi': None, 'last_updated': '2024-10-08T00:54:10.389Z'}, {'id': 'pyth-network', 'symbol': 'pyth', 'name': 'Pyth Network', 'image': 'https://coin-images.coingecko.com/coins/images/31924/large/pyth.png?1701245725', 'current_price': 0.327718, 'market_cap': 1187587206, 'market_cap_rank': 71, 'fully_diluted_valuation': 3276112770, 'total_volume': 71153373, 'high_24h': 0.347405, 'low_24h': 0.323434, 'price_change_24h': -0.014656647843092951, 'price_change_percentage_24h': -4.28087, 'market_cap_change_24h': -53646265.49825287, 'market_cap_change_percentage_24h': -4.32201, 'circulating_supply': 3624988786.43857, 'total_supply': 10000000000.0, 'max_supply': 10000000000.0, 'ath': 1.2, 'ath_change_percentage': -72.6067, 'ath_date': '2024-03-16T07:01:15.357Z', 'atl': 0.22352, 'atl_change_percentage': 46.61974, 'atl_date': '2024-08-05T11:30:50.894Z', 'roi': None, 'last_updated': '2024-10-08T00:54:11.687Z'}, {'id': 'helium', 'symbol': 'hnt', 'name': 'Helium', 'image': 'https://coin-images.coingecko.com/coins/images/4284/large/Helium_HNT.png?1696504894', 'current_price': 6.89, 'market_cap': 1175763601, 'market_cap_rank': 72, 'fully_diluted_valuation': 1535181838, 'total_volume': 21363150, 'high_24h': 7.5, 'low_24h': 6.8, 'price_change_24h': -0.5567727122519592, 'price_change_percentage_24h': -7.47186, 'market_cap_change_24h': -96629481.61365056, 'market_cap_change_percentage_24h': -7.59431, 'circulating_supply': 170791027.272522, 'total_supply': 223000000.0, 'max_supply': 223000000.0, 'ath': 54.88, 'ath_change_percentage': -87.4452, 'ath_date': '2021-11-12T23:08:25.301Z', 'atl': 0.113248, 'atl_change_percentage': 5983.74805, 'atl_date': '2020-04-18T00:19:10.902Z', 'roi': None, 'last_updated': '2024-10-08T00:54:08.888Z'}, {'id': 'solv-btc', 'symbol': 'solvbtc', 'name': 'Solv Protocol SolvBTC', 'image': 'https://coin-images.coingecko.com/coins/images/36800/large/solvBTC.png?1719810684', 'current_price': 62563, 'market_cap': 1166983495, 'market_cap_rank': 73, 'fully_diluted_valuation': 1166983495, 'total_volume': 4234286, 'high_24h': 64322, 'low_24h': 62260, 'price_change_24h': -972.2084470276677, 'price_change_percentage_24h': -1.5302, 'market_cap_change_24h': -18407428.421874046, 'market_cap_change_percentage_24h': -1.55286, 'circulating_supply': 18649.035274833, 'total_supply': 18649.035274833, 'max_supply': 21000000.0, 'ath': 70898, 'ath_change_percentage': -11.66845, 'ath_date': '2024-06-05T16:15:24.629Z', 'atl': 49058, 'atl_change_percentage': 27.6557, 'atl_date': '2024-08-05T06:28:45.311Z', 'roi': None, 'last_updated': '2024-10-08T00:53:36.145Z'}, {'id': 'celestia', 'symbol': 'tia', 'name': 'Celestia', 'image': 'https://coin-images.coingecko.com/coins/images/31967/large/tia.jpg?1696530772', 'current_price': 5.39, 'market_cap': 1160718301, 'market_cap_rank': 74, 'fully_diluted_valuation': 5799974465, 'total_volume': 223647640, 'high_24h': 5.72, 'low_24h': 5.33, 'price_change_24h': -0.03489822745982085, 'price_change_percentage_24h': -0.64286, 'market_cap_change_24h': -4896667.941010714, 'market_cap_change_percentage_24h': -0.42009, 'circulating_supply': 214906541.448367, 'total_supply': 1073863013.69837, 'max_supply': None, 'ath': 20.85, 'ath_change_percentage': -74.13297, 'ath_date': '2024-02-10T14:30:02.495Z', 'atl': 2.08, 'atl_change_percentage': 158.86734, 'atl_date': '2023-10-31T15:14:31.951Z', 'roi': None, 'last_updated': '2024-10-08T00:54:05.796Z'}, {'id': 'gatechain-token', 'symbol': 'gt', 'name': 'Gate', 'image': 'https://coin-images.coingecko.com/coins/images/8183/large/gate.png?1696508395', 'current_price': 8.86, 'market_cap': 1133702266, 'market_cap_rank': 75, 'fully_diluted_valuation': 2655869390, 'total_volume': 3255449, 'high_24h': 9.0, 'low_24h': 8.73, 'price_change_24h': 0.123653, 'price_change_percentage_24h': 1.4158, 'market_cap_change_24h': 15515512, 'market_cap_change_percentage_24h': 1.38756, 'circulating_supply': 128060017.17436442, 'total_supply': 300000000.0, 'max_supply': None, 'ath': 12.94, 'ath_change_percentage': -31.4915, 'ath_date': '2021-05-12T11:39:16.531Z', 'atl': 0.25754, 'atl_change_percentage': 3342.6755, 'atl_date': '2020-03-13T02:18:02.481Z', 'roi': None, 'last_updated': '2024-10-08T00:54:07.598Z'}, {'id': 'jupiter-exchange-solana', 'symbol': 'jup', 'name': 'Jupiter', 'image': 'https://coin-images.coingecko.com/coins/images/34188/large/jup.png?1704266489', 'current_price': 0.772541, 'market_cap': 1043006766, 'market_cap_rank': 76, 'fully_diluted_valuation': 7725976046, 'total_volume': 151655748, 'high_24h': 0.813931, 'low_24h': 0.767147, 'price_change_24h': -0.02900773798361378, 'price_change_percentage_24h': -3.61896, 'market_cap_change_24h': -38808691.17161238, 'market_cap_change_percentage_24h': -3.58737, 'circulating_supply': 1350000000.0, 'total_supply': 10000000000.0, 'max_supply': 10000000000.0, 'ath': 2.0, 'ath_change_percentage': -61.2806, 'ath_date': '2024-01-31T15:02:47.304Z', 'atl': 0.457464, 'atl_change_percentage': 69.27956, 'atl_date': '2024-02-21T18:31:05.083Z', 'roi': None, 'last_updated': '2024-10-08T00:54:09.568Z'}, {'id': 'algorand', 'symbol': 'algo', 'name': 'Algorand', 'image': 'https://coin-images.coingecko.com/coins/images/4380/large/download.png?1696504978', 'current_price': 0.125351, 'market_cap': 1039769409, 'market_cap_rank': 77, 'fully_diluted_valuation': 1039769414, 'total_volume': 57158385, 'high_24h': 0.129693, 'low_24h': 0.124391, 'price_change_24h': -0.002723524453178167, 'price_change_percentage_24h': -2.12651, 'market_cap_change_24h': -20685980.82075572, 'market_cap_change_percentage_24h': -1.95067, 'circulating_supply': 8292746539.53288, 'total_supply': 8292746578.70868, 'max_supply': 10000000000.0, 'ath': 3.56, 'ath_change_percentage': -96.48322, 'ath_date': '2019-06-20T14:51:19.480Z', 'atl': 0.087513, 'atl_change_percentage': 43.10684, 'atl_date': '2023-09-11T19:42:08.247Z', 'roi': {'times': -0.9477703186223201, 'currency': 'usd', 'percentage': -94.77703186223201}, 'last_updated': '2024-10-08T00:54:06.162Z'}, {'id': 'matic-network', 'symbol': 'matic', 'name': 'Polygon', 'image': 'https://coin-images.coingecko.com/coins/images/4713/large/polygon.png?1698233745', 'current_price': 0.377139, 'market_cap': 1031513152, 'market_cap_rank': 78, 'fully_diluted_valuation': 3770427519, 'total_volume': 10428459, 'high_24h': 0.389223, 'low_24h': 0.375421, 'price_change_24h': -0.005050292179460625, 'price_change_percentage_24h': -1.32141, 'market_cap_change_24h': -1119194.28873837, 'market_cap_change_percentage_24h': -0.10838, 'circulating_supply': 2735798915.4468446, 'total_supply': 10000000000.0, 'max_supply': 10000000000.0, 'ath': 2.92, 'ath_change_percentage': -87.0595, 'ath_date': '2021-12-27T02:08:34.307Z', 'atl': 0.00314376, 'atl_change_percentage': 11904.06254, 'atl_date': '2019-05-10T00:00:00.000Z', 'roi': {'times': 142.3989391138943, 'currency': 'usd', 'percentage': 14239.89391138943}, 'last_updated': '2024-10-08T00:54:10.159Z'}, {'id': 'ondo-finance', 'symbol': 'ondo', 'name': 'Ondo', 'image': 'https://coin-images.coingecko.com/coins/images/26580/large/ONDO.png?1696525656', 'current_price': 0.711239, 'market_cap': 1020937000, 'market_cap_rank': 79, 'fully_diluted_valuation': 7106589499, 'total_volume': 233426961, 'high_24h': 0.767711, 'low_24h': 0.705277, 'price_change_24h': -0.0452137306854673, 'price_change_percentage_24h': -5.97708, 'market_cap_change_24h': -67890921.7707479, 'market_cap_change_percentage_24h': -6.23523, 'circulating_supply': 1436606124.1074963, 'total_supply': 10000000000.0, 'max_supply': 10000000000.0, 'ath': 1.48, 'ath_change_percentage': -51.93391, 'ath_date': '2024-06-03T08:29:55.744Z', 'atl': 0.082171, 'atl_change_percentage': 765.00038, 'atl_date': '2024-01-18T12:14:30.524Z', 'roi': None, 'last_updated': '2024-10-08T00:54:10.869Z'}, {'id': 'worldcoin-wld', 'symbol': 'wld', 'name': 'Worldcoin', 'image': 'https://coin-images.coingecko.com/coins/images/31069/large/worldcoin.jpeg?1696529903', 'current_price': 1.95, 'market_cap': 994535999, 'market_cap_rank': 80, 'fully_diluted_valuation': 19530175731, 'total_volume': 540964835, 'high_24h': 2.06, 'low_24h': 1.91, 'price_change_24h': -0.008271319197278306, 'price_change_percentage_24h': -0.42173, 'market_cap_change_24h': 4400790, 'market_cap_change_percentage_24h': 0.44446, 'circulating_supply': 509230440.396566, 'total_supply': 10000000000.0, 'max_supply': 10000000000.0, 'ath': 11.74, 'ath_change_percentage': -83.29774, 'ath_date': '2024-03-10T00:10:42.330Z', 'atl': 0.973104, 'atl_change_percentage': 101.51475, 'atl_date': '2023-09-13T07:36:44.064Z', 'roi': None, 'last_updated': '2024-10-08T00:53:49.245Z'}, {'id': 'quant-network', 'symbol': 'qnt', 'name': 'Quant', 'image': 'https://coin-images.coingecko.com/coins/images/3370/large/5ZOu7brX_400x400.jpg?1696504070', 'current_price': 67.87, 'market_cap': 986909088, 'market_cap_rank': 81, 'fully_diluted_valuation': 991544793, 'total_volume': 18537323, 'high_24h': 71.06, 'low_24h': 67.1, 'price_change_24h': -2.311314272384635, 'price_change_percentage_24h': -3.2935, 'market_cap_change_24h': -34028927.77297032, 'market_cap_change_percentage_24h': -3.3331, 'circulating_supply': 14544176.164091088, 'total_supply': 14612493.0, 'max_supply': 14612493.0, 'ath': 427.42, 'ath_change_percentage': -84.11226, 'ath_date': '2021-09-11T09:15:00.668Z', 'atl': 0.215773, 'atl_change_percentage': 31371.79506, 'atl_date': '2018-08-23T00:00:00.000Z', 'roi': {'times': 10.988597073984174, 'currency': 'eth', 'percentage': 1098.8597073984174}, 'last_updated': '2024-10-08T00:54:10.405Z'}, {'id': 'lido-dao', 'symbol': 'ldo', 'name': 'Lido DAO', 'image': 'https://coin-images.coingecko.com/coins/images/13573/large/Lido_DAO.png?1696513326', 'current_price': 1.079, 'market_cap': 965929778, 'market_cap_rank': 82, 'fully_diluted_valuation': 1078912610, 'total_volume': 116984366, 'high_24h': 1.14, 'low_24h': 1.07, 'price_change_24h': -0.02206092767483625, 'price_change_percentage_24h': -2.0029, 'market_cap_change_24h': -19209916.68047166, 'market_cap_change_percentage_24h': -1.94997, 'circulating_supply': 895280831.178504, 'total_supply': 1000000000.0, 'max_supply': 1000000000.0, 'ath': 7.3, 'ath_change_percentage': -85.19627, 'ath_date': '2021-08-20T08:35:20.158Z', 'atl': 0.40615, 'atl_change_percentage': 166.18197, 'atl_date': '2022-06-18T20:55:12.035Z', 'roi': None, 'last_updated': '2024-10-08T00:54:09.774Z'}, {'id': 'kucoin-shares', 'symbol': 'kcs', 'name': 'KuCoin', 'image': 'https://coin-images.coingecko.com/coins/images/1047/large/sa9z79.png?1696502152', 'current_price': 7.95, 'market_cap': 956761609, 'market_cap_rank': 83, 'fully_diluted_valuation': 1135548069, 'total_volume': 105920, 'high_24h': 8.13, 'low_24h': 7.88, 'price_change_24h': -0.01620126524070553, 'price_change_percentage_24h': -0.20344, 'market_cap_change_24h': -1863355.6644928455, 'market_cap_change_percentage_24h': -0.19438, 'circulating_supply': 120406971.14608, 'total_supply': 142906971.14608, 'max_supply': None, 'ath': 28.83, 'ath_change_percentage': -72.50285, 'ath_date': '2021-12-01T15:09:35.541Z', 'atl': 0.342863, 'atl_change_percentage': 2212.11774, 'atl_date': '2019-02-07T00:00:00.000Z', 'roi': None, 'last_updated': '2024-10-08T00:54:08.466Z'}, {'id': 'jasmycoin', 'symbol': 'jasmy', 'name': 'JasmyCoin', 'image': 'https://coin-images.coingecko.com/coins/images/13876/large/JASMY200x200.jpg?1696513620', 'current_price': 0.01934656, 'market_cap': 936477923, 'market_cap_rank': 84, 'fully_diluted_valuation': 967036269, 'total_volume': 96934539, 'high_24h': 0.02058099, 'low_24h': 0.01921798, 'price_change_24h': -0.000913663078247352, 'price_change_percentage_24h': -4.50964, 'market_cap_change_24h': -43929303.850437164, 'market_cap_change_percentage_24h': -4.48072, 'circulating_supply': 48419999999.3058, 'total_supply': 50000000000.0, 'max_supply': 50000000000.0, 'ath': 4.79, 'ath_change_percentage': -99.59613, 'ath_date': '2021-02-16T03:53:32.207Z', 'atl': 0.00275026, 'atl_change_percentage': 603.41823, 'atl_date': '2022-12-29T20:41:09.113Z', 'roi': None, 'last_updated': '2024-10-08T00:54:09.140Z'}, {'id': 'bitcoin-cash-sv', 'symbol': 'bsv', 'name': 'Bitcoin SV', 'image': 'https://coin-images.coingecko.com/coins/images/6799/large/BSV.png?1696507128', 'current_price': 45.98, 'market_cap': 908863334, 'market_cap_rank': 85, 'fully_diluted_valuation': 965558272, 'total_volume': 14295577, 'high_24h': 47.42, 'low_24h': 45.54, 'price_change_24h': -1.3404163753512677, 'price_change_percentage_24h': -2.83266, 'market_cap_change_24h': -26325157.159350753, 'market_cap_change_percentage_24h': -2.81496, 'circulating_supply': 19766937.5, 'total_supply': 21000000.0, 'max_supply': 21000000.0, 'ath': 489.75, 'ath_change_percentage': -90.62735, 'ath_date': '2021-04-16T17:09:04.630Z', 'atl': 21.43, 'atl_change_percentage': 114.14966, 'atl_date': '2023-06-10T04:32:12.266Z', 'roi': None, 'last_updated': '2024-10-08T00:54:05.350Z'}, {'id': 'conflux-token', 'symbol': 'cfx', 'name': 'Conflux', 'image': 'https://coin-images.coingecko.com/coins/images/13079/large/3vuYMbjN.png?1696512867', 'current_price': 0.198997, 'market_cap': 897133314, 'market_cap_rank': 87, 'fully_diluted_valuation': 1099701725, 'total_volume': 136203952, 'high_24h': 0.197642, 'low_24h': 0.181839, 'price_change_24h': 0.01636931, 'price_change_percentage_24h': 8.96323, 'market_cap_change_24h': 70613398, 'market_cap_change_percentage_24h': 8.54346, 'circulating_supply': 4526069775.50803, 'total_supply': 5548034679.73236, 'max_supply': None, 'ath': 1.7, 'ath_change_percentage': -88.39547, 'ath_date': '2021-03-27T03:43:35.178Z', 'atl': 0.02199898, 'atl_change_percentage': 797.20122, 'atl_date': '2022-12-30T08:16:30.345Z', 'roi': None, 'last_updated': '2024-10-08T00:53:33.560Z'}, {'id': 'bittorrent', 'symbol': 'btt', 'name': 'BitTorrent', 'image': 'https://coin-images.coingecko.com/coins/images/22457/large/btt_logo.png?1696521780', 'current_price': 9.23941e-07, 'market_cap': 894567068, 'market_cap_rank': 86, 'fully_diluted_valuation': 914665287, 'total_volume': 26689838, 'high_24h': 9.43491e-07, 'low_24h': 9.21746e-07, 'price_change_24h': -1.8515579683e-08, 'price_change_percentage_24h': -1.96461, 'market_cap_change_24h': -16353484.723588705, 'market_cap_change_percentage_24h': -1.79527, 'circulating_supply': 968246428571000.0, 'total_supply': 990000000000000.0, 'max_supply': 990000000000000.0, 'ath': 3.43e-06, 'ath_change_percentage': -73.05988, 'ath_date': '2022-01-21T04:00:31.909Z', 'atl': 3.65368e-07, 'atl_change_percentage': 153.00723, 'atl_date': '2023-10-13T05:10:41.241Z', 'roi': None, 'last_updated': '2024-10-08T00:54:05.559Z'}, {'id': 'based-brett', 'symbol': 'brett', 'name': 'Brett', 'image': 'https://coin-images.coingecko.com/coins/images/35529/large/1000050750.png?1709031995', 'current_price': 0.088115, 'market_cap': 873218316, 'market_cap_rank': 88, 'fully_diluted_valuation': 873218316, 'total_volume': 58537246, 'high_24h': 0.092752, 'low_24h': 0.085926, 'price_change_24h': 0.00054038, 'price_change_percentage_24h': 0.61706, 'market_cap_change_24h': 5528824, 'market_cap_change_percentage_24h': 0.63719, 'circulating_supply': 9910164240.66912, 'total_supply': 9910164240.66912, 'max_supply': 9999998988.0, 'ath': 0.193328, 'ath_change_percentage': -54.36865, 'ath_date': '2024-06-09T12:55:51.835Z', 'atl': 0.00084753, 'atl_change_percentage': 10308.88083, 'atl_date': '2024-02-29T08:40:24.951Z', 'roi': None, 'last_updated': '2024-10-08T00:54:05.104Z'}, {'id': 'coredaoorg', 'symbol': 'core', 'name': 'Core', 'image': 'https://coin-images.coingecko.com/coins/images/28938/large/file_589.jpg?1701868471', 'current_price': 0.937112, 'market_cap': 858110315, 'market_cap_rank': 89, 'fully_diluted_valuation': 1971753958, 'total_volume': 31657462, 'high_24h': 0.967114, 'low_24h': 0.932028, 'price_change_24h': -0.01422813882470342, 'price_change_percentage_24h': -1.49559, 'market_cap_change_24h': -10961374.97734642, 'market_cap_change_percentage_24h': -1.26127, 'circulating_supply': 913923187.211838, 'total_supply': 2100000000.0, 'max_supply': 2100000000.0, 'ath': 6.14, 'ath_change_percentage': -84.69421, 'ath_date': '2023-02-08T12:55:39.828Z', 'atl': 0.334237, 'atl_change_percentage': 181.33666, 'atl_date': '2023-11-03T01:20:55.441Z', 'roi': None, 'last_updated': '2024-10-08T00:54:07.590Z'}, {'id': 'fasttoken', 'symbol': 'ftn', 'name': 'Fasttoken', 'image': 'https://coin-images.coingecko.com/coins/images/28478/large/lightenicon_200x200.png?1696527472', 'current_price': 2.6, 'market_cap': 856233446, 'market_cap_rank': 90, 'fully_diluted_valuation': 2290629881, 'total_volume': 215412570, 'high_24h': 2.62, 'low_24h': 2.51, 'price_change_24h': 0.062821, 'price_change_percentage_24h': 2.47271, 'market_cap_change_24h': 21132723, 'market_cap_change_percentage_24h': 2.53056, 'circulating_supply': 328942461.97373456, 'total_supply': 880000000.0, 'max_supply': 1000000000.0, 'ath': 2.62, 'ath_change_percentage': -0.4686, 'ath_date': '2024-10-07T19:07:59.036Z', 'atl': 0.398142, 'atl_change_percentage': 553.93665, 'atl_date': '2023-01-21T10:15:39.503Z', 'roi': None, 'last_updated': '2024-10-08T00:54:08.935Z'}, {'id': 'gala', 'symbol': 'gala', 'name': 'GALA', 'image': 'https://coin-images.coingecko.com/coins/images/12493/large/GALA_token_image_-_200PNG.png?1709725869', 'current_price': 0.02149496, 'market_cap': 848757809, 'market_cap_rank': 91, 'fully_diluted_valuation': 848757654, 'total_volume': 161097632, 'high_24h': 0.02224224, 'low_24h': 0.02072146, 'price_change_24h': 0.00021638, 'price_change_percentage_24h': 1.01687, 'market_cap_change_24h': 9005263, 'market_cap_change_percentage_24h': 1.07237, 'circulating_supply': 39481499168.913, 'total_supply': 39481491922.3056, 'max_supply': 50000000000.0, 'ath': 0.824837, 'ath_change_percentage': -97.39249, 'ath_date': '2021-11-26T01:03:48.731Z', 'atl': 0.00013475, 'atl_change_percentage': 15861.25537, 'atl_date': '2020-12-28T08:46:48.367Z', 'roi': None, 'last_updated': '2024-10-08T00:54:09.522Z'}, {'id': 'ether-fi-staked-eth', 'symbol': 'eeth', 'name': 'ether.fi Staked ETH', 'image': 'https://coin-images.coingecko.com/coins/images/33049/large/ether.fi_eETH.png?1700473063', 'current_price': 2424.85, 'market_cap': 846755524, 'market_cap_rank': 92, 'fully_diluted_valuation': 4871591578, 'total_volume': 106209, 'high_24h': 2508.25, 'low_24h': 2415.47, 'price_change_24h': -41.29035543839427, 'price_change_percentage_24h': -1.67429, 'market_cap_change_24h': -18371264.200617433, 'market_cap_change_percentage_24h': -2.12353, 'circulating_supply': 349139.81169378624, 'total_supply': 2008686.70827969, 'max_supply': None, 'ath': 5307.23, 'ath_change_percentage': -54.27135, 'ath_date': '2024-08-06T16:16:30.007Z', 'atl': 2155.76, 'atl_change_percentage': 12.57876, 'atl_date': '2024-02-08T11:26:30.368Z', 'roi': None, 'last_updated': '2024-10-08T00:54:07.140Z'}, {'id': 'wormhole', 'symbol': 'w', 'name': 'Wormhole', 'image': 'https://coin-images.coingecko.com/coins/images/35087/large/womrhole_logo_full_color_rgb_2000px_72ppi_fb766ac85a.png?1708688954', 'current_price': 0.324805, 'market_cap': 836255956, 'market_cap_rank': 93, 'fully_diluted_valuation': 3243975804, 'total_volume': 219924753, 'high_24h': 0.35574, 'low_24h': 0.323118, 'price_change_24h': -0.02045301877589417, 'price_change_percentage_24h': -5.92398, 'market_cap_change_24h': -51338984.688058615, 'market_cap_change_percentage_24h': -5.78406, 'circulating_supply': 2577873594.0, 'total_supply': 10000000000.0, 'max_supply': 10000000000.0, 'ath': 1.66, 'ath_change_percentage': -80.38595, 'ath_date': '2024-04-03T11:46:35.308Z', 'atl': 0.16434, 'atl_change_percentage': 97.84984, 'atl_date': '2024-08-05T06:26:45.610Z', 'roi': None, 'last_updated': '2024-10-08T00:53:48.826Z'}, {'id': 'flow', 'symbol': 'flow', 'name': 'Flow', 'image': 'https://coin-images.coingecko.com/coins/images/13446/large/5f6294c0c7a8cda55cb1c936_Flow_Wordmark.png?1696513210', 'current_price': 0.540995, 'market_cap': 831299188, 'market_cap_rank': 94, 'fully_diluted_valuation': 831299188, 'total_volume': 58056753, 'high_24h': 0.561971, 'low_24h': 0.53686, 'price_change_24h': -0.01656192450478211, 'price_change_percentage_24h': -2.97044, 'market_cap_change_24h': -24892946.25119114, 'market_cap_change_percentage_24h': -2.9074, 'circulating_supply': 1536086728.20855, 'total_supply': 1536086728.20855, 'max_supply': None, 'ath': 42.4, 'ath_change_percentage': -98.72532, 'ath_date': '2021-04-05T13:49:10.098Z', 'atl': 0.391969, 'atl_change_percentage': 37.87491, 'atl_date': '2023-09-11T19:41:06.528Z', 'roi': None, 'last_updated': '2024-10-08T00:54:08.411Z'}, {'id': 'notcoin', 'symbol': 'not', 'name': 'Notcoin', 'image': 'https://coin-images.coingecko.com/coins/images/33453/large/rFmThDiD_400x400.jpg?1701876350', 'current_price': 0.00801109, 'market_cap': 822138290, 'market_cap_rank': 95, 'fully_diluted_valuation': 822138290, 'total_volume': 264338630, 'high_24h': 0.00839936, 'low_24h': 0.00772693, 'price_change_24h': 0.00011801, 'price_change_percentage_24h': 1.49515, 'market_cap_change_24h': 13636202, 'market_cap_change_percentage_24h': 1.6866, 'circulating_supply': 102474422538.643, 'total_supply': 102474422538.643, 'max_supply': None, 'ath': 0.02836145, 'ath_change_percentage': -71.61676, 'ath_date': '2024-06-02T18:00:38.587Z', 'atl': 0.00461057, 'atl_change_percentage': 74.59678, 'atl_date': '2024-05-24T07:12:14.147Z', 'roi': None, 'last_updated': '2024-10-08T00:54:11.088Z'}, {'id': 'beam-2', 'symbol': 'beam', 'name': 'Beam', 'image': 'https://coin-images.coingecko.com/coins/images/32417/large/chain-logo.png?1698114384', 'current_price': 0.01552574, 'market_cap': 801595531, 'market_cap_rank': 96, 'fully_diluted_valuation': 968773026, 'total_volume': 39916618, 'high_24h': 0.01674658, 'low_24h': 0.01538882, 'price_change_24h': -0.000940684073936141, 'price_change_percentage_24h': -5.71274, 'market_cap_change_24h': -47924263.662335515, 'market_cap_change_percentage_24h': -5.64134, 'circulating_supply': 51660007768.0, 'total_supply': 62434008330.0, 'max_supply': 62434008330.0, 'ath': 0.04416304, 'ath_change_percentage': -64.79328, 'ath_date': '2024-03-10T10:40:22.381Z', 'atl': 0.0043383, 'atl_change_percentage': 258.39735, 'atl_date': '2023-10-29T08:20:14.064Z', 'roi': None, 'last_updated': '2024-10-08T00:54:13.203Z'}, {'id': 'renzo-restaked-eth', 'symbol': 'ezeth', 'name': 'Renzo Restaked ETH', 'image': 'https://coin-images.coingecko.com/coins/images/34753/large/Ezeth_logo_circle.png?1713496404', 'current_price': 2482.56, 'market_cap': 794760666, 'market_cap_rank': 97, 'fully_diluted_valuation': 794760666, 'total_volume': 11266087, 'high_24h': 2562.78, 'low_24h': 2455.06, 'price_change_24h': -51.8338887688119, 'price_change_percentage_24h': -2.04522, 'market_cap_change_24h': -22560571.011731625, 'market_cap_change_percentage_24h': -2.76031, 'circulating_supply': 320078.067454777, 'total_supply': 320078.067454777, 'max_supply': None, 'ath': 4106.74, 'ath_change_percentage': -39.47159, 'ath_date': '2024-03-12T00:44:29.429Z', 'atl': 2198.04, 'atl_change_percentage': 13.08921, 'atl_date': '2024-01-26T08:09:59.848Z', 'roi': None, 'last_updated': '2024-10-08T00:54:10.315Z'}, {'id': 'ethena', 'symbol': 'ena', 'name': 'Ethena', 'image': 'https://coin-images.coingecko.com/coins/images/36530/large/ethena.png?1711701436', 'current_price': 0.287191, 'market_cap': 789001209, 'market_cap_rank': 98, 'fully_diluted_valuation': 4308539023, 'total_volume': 209536876, 'high_24h': 0.311956, 'low_24h': 0.283762, 'price_change_24h': -0.013023047337344384, 'price_change_percentage_24h': -4.33793, 'market_cap_change_24h': -35648060.95506203, 'market_cap_change_percentage_24h': -4.32281, 'circulating_supply': 2746875000.0, 'total_supply': 15000000000.0, 'max_supply': None, 'ath': 1.52, 'ath_change_percentage': -81.04188, 'ath_date': '2024-04-11T13:15:15.057Z', 'atl': 0.195078, 'atl_change_percentage': 47.4937, 'atl_date': '2024-09-06T21:04:48.253Z', 'roi': None, 'last_updated': '2024-10-08T00:54:04.226Z'}, {'id': 'klay-token', 'symbol': 'klay', 'name': 'Klaytn', 'image': 'https://coin-images.coingecko.com/coins/images/9672/large/klaytn.png?1696509742', 'current_price': 0.132756, 'market_cap': 770707685, 'market_cap_rank': 99, 'fully_diluted_valuation': 774944601, 'total_volume': 15981912, 'high_24h': 0.13638, 'low_24h': 0.131797, 'price_change_24h': -0.002995926554336559, 'price_change_percentage_24h': -2.20692, 'market_cap_change_24h': -19069806.84438181, 'market_cap_change_percentage_24h': -2.41458, 'circulating_supply': 5806693656.94806, 'total_supply': 5838615577.49937, 'max_supply': None, 'ath': 4.34, 'ath_change_percentage': -96.93899, 'ath_date': '2021-03-30T03:44:28.828Z', 'atl': 0.06044, 'atl_change_percentage': 119.7475, 'atl_date': '2020-04-29T08:19:34.574Z', 'roi': None, 'last_updated': '2024-10-08T00:54:11.218Z'}, {'id': 'aerodrome-finance', 'symbol': 'aero', 'name': 'Aerodrome Finance', 'image': 'https://coin-images.coingecko.com/coins/images/31745/large/token.png?1696530564', 'current_price': 1.2, 'market_cap': 769656411, 'market_cap_rank': 100, 'fully_diluted_valuation': 1557648682, 'total_volume': 55612141, 'high_24h': 1.27, 'low_24h': 1.19, 'price_change_24h': -0.0154197910139926, 'price_change_percentage_24h': -1.26784, 'market_cap_change_24h': -9478210.726666927, 'market_cap_change_percentage_24h': -1.2165, 'circulating_supply': 641571846.36209, 'total_supply': 1298428138.12827, 'max_supply': None, 'ath': 2.31, 'ath_change_percentage': -48.0214, 'ath_date': '2024-04-12T03:44:52.080Z', 'atl': 1.861e-05, 'atl_change_percentage': 6440257.63163, 'atl_date': '2023-10-17T01:23:50.860Z', 'roi': None, 'last_updated': '2024-10-08T00:54:07.760Z'}]
# Loop through the data and print the name and current price
for coin in data:
= coin['name']
name = coin['current_price']
price print(f"Coin: {name}, Price: ${price}")
Coin: Bitcoin, Price: $62490
Coin: Ethereum, Price: $2434.1
Coin: Tether, Price: $0.999711
Coin: BNB, Price: $568.59
Coin: Solana, Price: $144.62
Coin: USDC, Price: $0.99982
Coin: XRP, Price: $0.531761
Coin: Lido Staked Ether, Price: $2433.54
Coin: Dogecoin, Price: $0.109138
Coin: TRON, Price: $0.156189
Coin: Toncoin, Price: $5.23
Coin: Cardano, Price: $0.35311
Coin: Avalanche, Price: $26.86
Coin: Shiba Inu, Price: $1.759e-05
Coin: Wrapped stETH, Price: $2870.49
Coin: Wrapped Bitcoin, Price: $62339
Coin: WETH, Price: $2434.35
Coin: Chainlink, Price: $11.22
Coin: Bitcoin Cash, Price: $325.66
Coin: Polkadot, Price: $4.15
Coin: Dai, Price: $0.999811
Coin: Sui, Price: $2.06
Coin: NEAR Protocol, Price: $5.11
Coin: LEO Token, Price: $6.01
Coin: Uniswap, Price: $7.24
Coin: Litecoin, Price: $65.04
Coin: Bittensor, Price: $617.07
Coin: Aptos, Price: $8.91
Coin: Pepe, Price: $9.89e-06
Coin: Wrapped eETH, Price: $2554.88
Coin: Artificial Superintelligence Alliance, Price: $1.49
Coin: Internet Computer, Price: $8.13
Coin: Kaspa, Price: $0.137693
Coin: POL (ex-MATIC), Price: $0.376926
Coin: Ethereum Classic, Price: $18.7
Coin: Stellar, Price: $0.091505
Coin: Monero, Price: $144.06
Coin: Stacks, Price: $1.77
Coin: First Digital USD, Price: $0.999501
Coin: dogwifhat, Price: $2.56
Coin: OKB, Price: $41.75
Coin: Ethena USDe, Price: $0.998868
Coin: Immutable, Price: $1.49
Coin: Filecoin, Price: $3.74
Coin: Aave, Price: $146.68
Coin: Cronos, Price: $0.078844
Coin: Optimism, Price: $1.67
Coin: Render, Price: $5.32
Coin: Injective, Price: $20.63
Coin: Arbitrum, Price: $0.55172
Coin: Hedera, Price: $0.052714
Coin: Mantle, Price: $0.594723
Coin: Fantom, Price: $0.680296
Coin: VeChain, Price: $0.02305745
Coin: Cosmos Hub, Price: $4.44
Coin: THORChain, Price: $5.09
Coin: WhiteBIT Coin, Price: $11.61
Coin: The Graph, Price: $0.16643
Coin: Sei, Price: $0.436364
Coin: Bitget Token, Price: $1.075
Coin: Bonk, Price: $2.134e-05
Coin: Binance-Peg WETH, Price: $2434.55
Coin: FLOKI, Price: $0.00013831
Coin: Rocket Pool ETH, Price: $2719.44
Coin: Theta Network, Price: $1.31
Coin: Popcat, Price: $1.28
Coin: Arweave, Price: $19.03
Coin: Maker, Price: $1406.89
Coin: Mantle Staked Ether, Price: $2540.6
Coin: MANTRA, Price: $1.4
Coin: Pyth Network, Price: $0.327718
Coin: Helium, Price: $6.89
Coin: Solv Protocol SolvBTC, Price: $62563
Coin: Celestia, Price: $5.39
Coin: Gate, Price: $8.86
Coin: Jupiter, Price: $0.772541
Coin: Algorand, Price: $0.125351
Coin: Polygon, Price: $0.377139
Coin: Ondo, Price: $0.711239
Coin: Worldcoin, Price: $1.95
Coin: Quant, Price: $67.87
Coin: Lido DAO, Price: $1.079
Coin: KuCoin, Price: $7.95
Coin: JasmyCoin, Price: $0.01934656
Coin: Bitcoin SV, Price: $45.98
Coin: Conflux, Price: $0.198997
Coin: BitTorrent, Price: $9.23941e-07
Coin: Brett, Price: $0.088115
Coin: Core, Price: $0.937112
Coin: Fasttoken, Price: $2.6
Coin: GALA, Price: $0.02149496
Coin: ether.fi Staked ETH, Price: $2424.85
Coin: Wormhole, Price: $0.324805
Coin: Flow, Price: $0.540995
Coin: Notcoin, Price: $0.00801109
Coin: Beam, Price: $0.01552574
Coin: Renzo Restaked ETH, Price: $2482.56
Coin: Ethena, Price: $0.287191
Coin: Klaytn, Price: $0.132756
Coin: Aerodrome Finance, Price: $1.2
5.6 Independent Study
5.6.1 Practice exercise 1: Reading .csv
data
Read the file Top 10 Albums By Year.csv. This file contains the top 10 albums for each year from 1990 to 2021. Each row corresponds to a unique album.
5.6.1.1
Print the first 5 rows of the data.
5.6.1.2
How many rows and columns are there in the data?
5.6.1.3
Print the summary statistics of the data, and answer the following questions:
- What proportion of albums have 15 or lesser tracks? Mention a range for the proportion.
- What is the mean length of a track (in minutes)?
5.6.1.4
Find the album having the highest worldwide sales per year, and its artist.
5.6.1.5
Subset the data to include only Hip-Hop albums. How many Hip_Hop albums are there?
5.6.1.6
Which album amongst hip-hop has the higest mean sales per year per track, and who is its artist?
5.6.2 Practice exercise 2: Reading .txt
data
Read the file bestseller_books.txt. It contains top 50 best-selling books on amazon from 2009 to 2019. Identify the delimiter without opening the file with Notepad or a text-editing software. How many rows and columns are there in the dataset?
Solution:
#The delimiter seems to be ';' based on the output of the above code
= pd.read_csv('../Data/bestseller_books.txt',sep=';')
bestseller_books bestseller_books.head()
Unnamed: 0.1 | Unnamed: 0 | Name | Author | User Rating | Reviews | Price | Year | Genre | |
---|---|---|---|---|---|---|---|---|---|
0 | 0 | 0 | 10-Day Green Smoothie Cleanse | JJ Smith | 4.7 | 17350 | 8 | 2016 | Non Fiction |
1 | 1 | 1 | 11/22/63: A Novel | Stephen King | 4.6 | 2052 | 22 | 2011 | Fiction |
2 | 2 | 2 | 12 Rules for Life: An Antidote to Chaos | Jordan B. Peterson | 4.7 | 18979 | 15 | 2018 | Non Fiction |
3 | 3 | 3 | 1984 (Signet Classics) | George Orwell | 4.7 | 21424 | 6 | 2017 | Fiction |
4 | 4 | 4 | 5,000 Awesome Facts (About Everything!) (Natio... | National Geographic Kids | 4.8 | 7665 | 12 | 2019 | Non Fiction |
#The file read with ';' as the delimited is correct
print("The file has",bestseller_books.shape[0],"rows and",bestseller_books.shape[1],"columns")
The file has 550 rows and 9 columns
Alternatively, you can use the argument sep = None
, and engine = 'python'
. The default engine is C. However, the ‘python’ engine has a ‘sniffer’ tool which may identify the delimiter automatically.
= pd.read_csv('../data/bestseller_books.txt',sep=None, engine = 'python')
bestseller_books bestseller_books.head()
Unnamed: 0.1 | Unnamed: 0 | Name | Author | User Rating | Reviews | Price | Year | Genre | |
---|---|---|---|---|---|---|---|---|---|
0 | 0 | 0 | 10-Day Green Smoothie Cleanse | JJ Smith | 4.7 | 17350 | 8 | 2016 | Non Fiction |
1 | 1 | 1 | 11/22/63: A Novel | Stephen King | 4.6 | 2052 | 22 | 2011 | Fiction |
2 | 2 | 2 | 12 Rules for Life: An Antidote to Chaos | Jordan B. Peterson | 4.7 | 18979 | 15 | 2018 | Non Fiction |
3 | 3 | 3 | 1984 (Signet Classics) | George Orwell | 4.7 | 21424 | 6 | 2017 | Fiction |
4 | 4 | 4 | 5,000 Awesome Facts (About Everything!) (Natio... | National Geographic Kids | 4.8 | 7665 | 12 | 2019 | Non Fiction |
5.6.3 Practice exercise 3: Reading HTML data
Read the table(s) consisting of attendance of spectators in FIFA worlds cup from this page. Read only those table(s) that have the word ‘attendance’ in them. How many rows and columns are there in the table(s)?
= pd.read_html('https://en.wikipedia.org/wiki/FIFA_World_Cup',
dfs ='reaching')
matchprint(len(dfs))
= dfs[0]
data print("Number of rows =",data.shape[0], "and number of columns=",data.shape[1])
data.head()
1
Number of rows = 25 and number of columns= 6
Team | Titles | Runners-up | Third place | Fourth place | Top 4 total | |
---|---|---|---|---|---|---|
0 | Brazil | 5 (1958, 1962, 1970, 1994, 2002) | 2 (1950 *, 1998) | 2 (1938, 1978) | 2 (1974, 2014 *) | 11 |
1 | Germany1 | 4 (1954, 1974 *, 1990, 2014) | 4 (1966, 1982, 1986, 2002) | 4 (1934, 1970, 2006 *, 2010) | 1 (1958) | 13 |
2 | Italy | 4 (1934 *, 1938, 1982, 2006) | 2 (1970, 1994) | 1 (1990 *) | 1 (1978) | 8 |
3 | Argentina | 3 (1978 *, 1986, 2022) | 3 (1930, 1990, 2014) | NaN | NaN | 6 |
4 | France | 2 (1998 *, 2018) | 2 (2006, 2022) | 2 (1958, 1986) | 1 (1982) | 7 |
5.6.4 Practice exercise 4: Reading JSON data
Read the movies dataset from here. How many rows and columns are there in the data?
= pd.read_json('https://raw.githubusercontent.com/vega/vega-datasets/master/data/movies.json')
movies_data print("Number of rows =",movies_data.shape[0], "and number of columns=",movies_data.shape[1])
Number of rows = 3201 and number of columns= 16